An easy yet interesting project which can be done within a day in Python

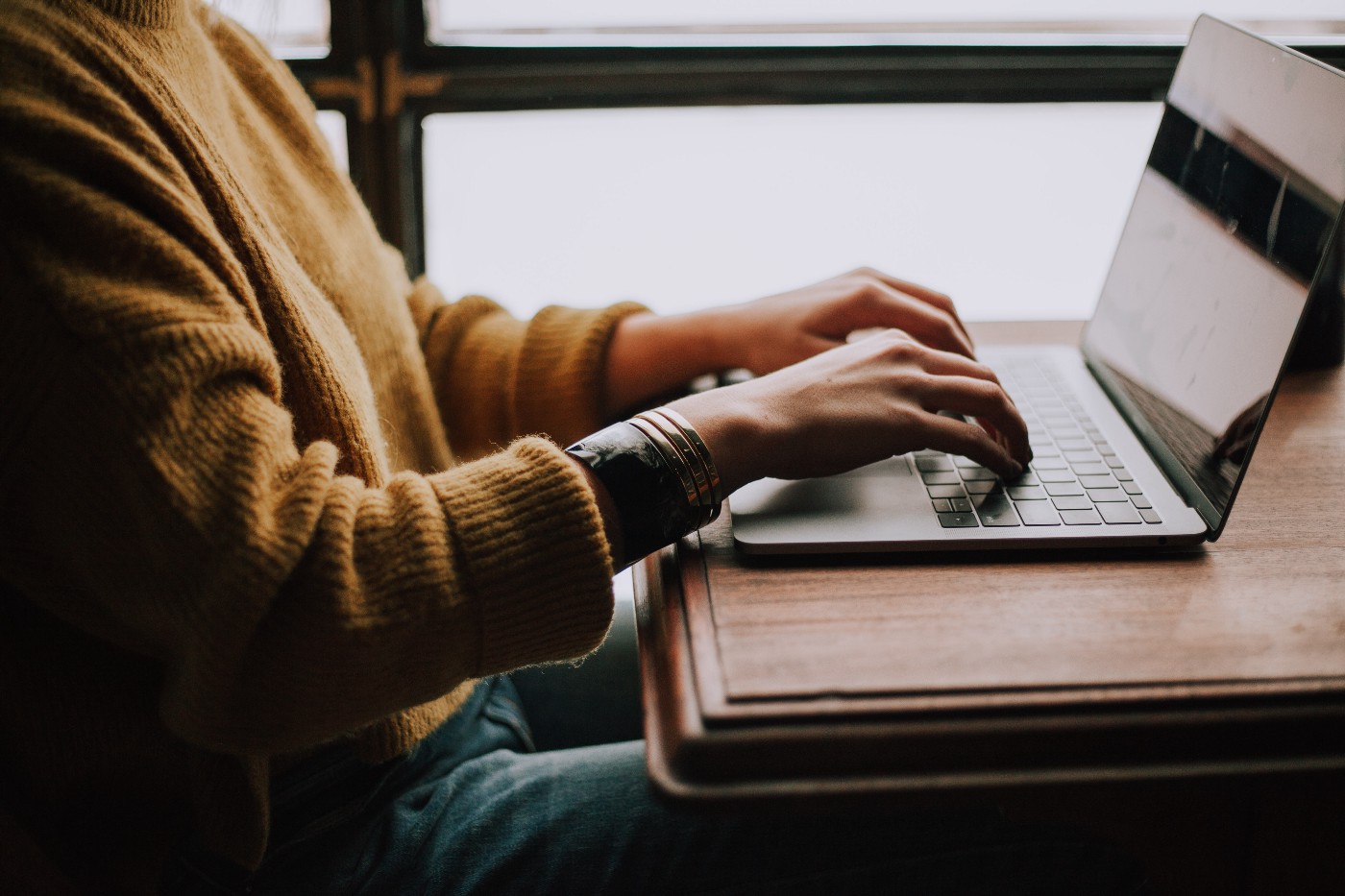
The most important thing I understood while learning to code is that none other than projects could teach you the real-world stuff you need to know. And projects are the most interesting aspects of programming as you can create what you imagine. I’ve been in the stock market space for a while from now and since I know Python, I took to creating a lot of stock-based programming projects.
In this article, I’m going to walk you through one of my most simple yet interesting stock market projects which utilize the search API provided by EOD Historical Data (EODhd) to build a search application and allows the users to search for different stocks along with other additional types of assets. Without further ado, let’s dive into the article!
Note: This article is intensely based on the APIs provided by EOD Historical Data. So to follow up with the upcoming content without any distress, make sure that you have an account for yourself as it enables you to access your private API key. You can create an account using the link here. Also, not all APIs mentioned in this article are offered for free but a subscription is needed. View the pricing of all subscriptions here and choose what best fits you.
1. Importing Packages
Let’s first start by importing the required packages into our python environment as it is the foremost process in any programming project. The only packages we need in this article are Requests to make API calls and Pandas for working with the data. In case you have not yet installed these two packages, enter the following lines of code in your command line:
pip install requests
pip install pandas
After installing the packages, we can now import them into our python environment using the code below:
import requests
import pandas as pdapi_key = 'YOUR API KEY'
We have now successfully imported the essential packages into our environment along with storing the API key into the api_key
variable.
2. Creating the Search Function
In this step, we are going to define a function that searches for assets based on the given inputs and returns a dataframe that comprises the search results of the asset along with other market information. The function might sound to hold a lot of functionality but can be easily programmed in Python and the code would look something like this:
def search_asset(asset, search_input, api_key):
url = f'https://eodhistoricaldata.com/api/search/{search_input}?api_token={api_key}&type={asset}'
results_json = requests.get(url).json()
results_df = pd.DataFrame(results_json).drop('ISIN', axis = 1).rename(columns = {'Code':'Symbol'})return results_df
Now let’s break down the above-shown code snippet. The first thing we are doing is to define a function named search_asset
that takes the type of asset (asset
), the name of the asset we want to run the search for (search_input
), and the API key (api_key
) as parameters.
Inside the function, we are first storing the API URL into the url
variable and using the get
function provided by the Requests packages, we are making an API call to extract the data. Before proceeding any further, let’s spare some time to take a look at the API URL and analyze its structure:
https://eodhistoricaldata.com/api/search/{search_input}?api_token={api_key}&type={asset}
The first and foremost aspect we have to look at in the above URL is the query_string
that comes after /search
where we have to specify the name of the asset we want to search for, it can be a company name or ticker.
Next, there are two parameters: the first being the api_token
parameter where the API key goes in, and the second being the type
parameter where we have to mention the type of asset we are looking for (available assets: stock, ETF, fund, bonds, index, commodity, cryptocurrency). There are several different parameters apart from the ones used here which can be used for further filtering of the data but these two are enough for our application.
Coming back to the code, after making an API call and storing the fetched data, we are converting the JSON data into a Pandas dataframe to make more sense out of it and we are also doing some data manipulations to clean it, and finally, we are returning the dataframe.
3. Extracting Inputs and Showing the Results
The next and final step of this project is to get the necessary inputs from the user and use those to feed into the function we created before to show the final results. For this app, we will extract two different inputs: type of the asset (stock, ETF, bonds, cryptocurrency, etc.), and the name of the asset to search for.
Now, there are several different ways of getting inputs from the user in Python but the most basic and efficient way is to make use of the input
function which is a built-in feature in Python. The code for getting the right inputs for our app and showing the final results look like this:
assets = pd.DataFrame(pd.Series(['stock', 'etf', 'fund', 'bonds', 'index', 'commodity', 'crypto'])).rename(columns = {0:'asset'})
asset = input('Type of asset [stock, etf, fund, bonds, index, commodity, crypto]: ')if len(assets[assets.asset == asset]) == 0:
print(cl('Input Error: Asset not found', color = 'red'))
else:
search_input = input(f'Search {asset}: ')
print(cl(f'nSearch Results for {search_input}', attrs = ['bold']), 'n')
search_results = search_asset(asset, search_input, api_key)
display(search_results)
In the above code, we are first creating a dataframe that comprises all the available assets. Next, we are creating an input field to get what type of asset the user is looking for using the built-in input
function and the input extracted from the user will be stored into the asset
variable.
Then, we are creating an if-statement that spits out an error message if the user types something random or an asset that is not available. But when the input extracted is looking fine, the if-statement will proceed to get the next type of input from the user which is the asset name they want to run the search for.
After extracting the required inputs, we are feeding those into the search_asset
function we created before to run the search and the final results are stored into the search_results
variable followed by representing the dataframe using the display
provided by the Pandas package. Here is an example of searching for stocks using the app we created:
In this article, we learned how can a simple search application can be constructed using EODhd’s Search API and there is also plenty of room for improvement.
One interesting way you can do to take this project to the next level is by using the search function we created previously in this article as the base function to run a search based on the inputs and represent the real-time data pulled using EODhd’s Real-Time APIs of the asset along with some intriguing charts and visuals, and ultimately turn that into a dashboard.
The most important thing to be noted in this article is that it took not less than twenty-five lines of code to build the whole application in Python and this is mainly because of the Search API provided by EODhd that helped oversimply the process of extracting the search results. Without that, we would have to undergo even more programming steps in vain but thanks to the existing powerful APIs, we now don’t necessarily need that.
With that being said, you’ve reached the end of the article. Hope you learned something new and useful. Also, if you want to see the original documentation for more information, view it using this link: https://eodhistoricaldata.com/financial-apis/search-api-for-stocks-etfs-mutual-funds-and-indices/