A hands-on tutorial on using EOD Historical Data’s API for financial news

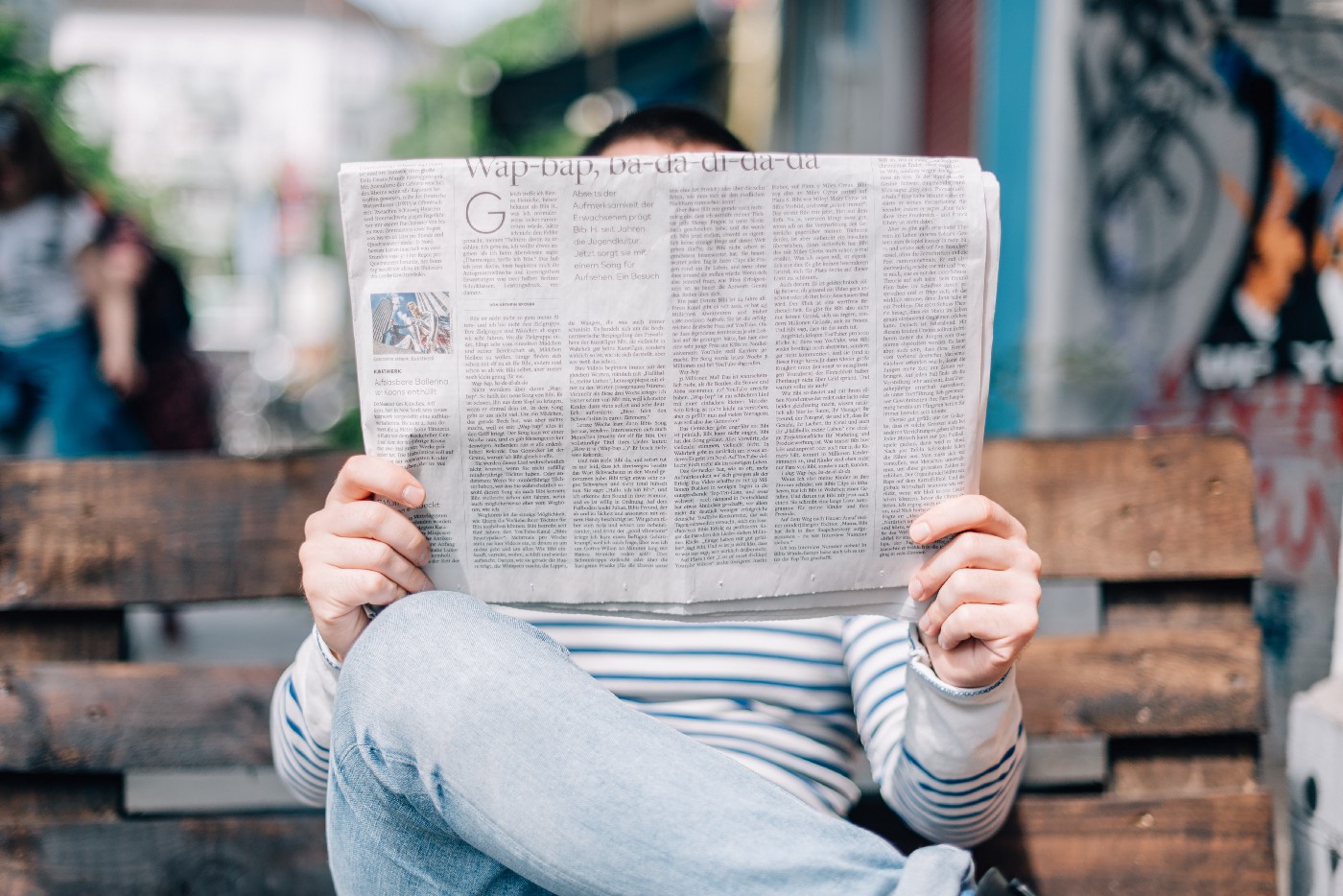
Table of Contents:
- Introduction
- Extracting Financial News with Python
-- Importing Packages
-- Basic Program for Extracting Financial News
-- Advanced Program for Extracting Financial News
- Closing Notes
It is essential for an investor to keep himself updated about the markets in order to secure maximum advantage. Standard newspapers specializing in finance and investments are a good source and more than enough for long-term investors or people who are investing in the market as a stream of passive income. But, day traders have to know what’s happening around them on a real-time basis given the tough competitive environment.
Though there are a lot of resources for real-time news, it either bounds to be expensive or lacks customization. So the best route, according to me, is automating the process with Python using APIs but the problem is, all APIs are not reliable. But EOD Historical Data is here to save us with their powerful financial news APIs which are flexible and more importantly reliable. In this article. I’m going to walk you through the whole process of extracting financial news using EOD Historical Data’s APIs with Python. Let’s dive into it!
Note: This article is intensely based on the APIs provided by EOD Historical Data. So to follow up with the upcoming content without any distress, make sure that you have an account for yourself as it enables you to access your private API key. You can create an account using the link here. Also, not all APIs mentioned in this article is offered for free but a subscription is needed. View the pricing of all subscriptions here and choose what best fits you.
1. Importing Packages
Let’s first start by importing the required packages into our python environment as it is the foremost process in any programming project. The only packages we need in this article are Requests to make API calls and Termcolor for font or text customization. In case you have not yet installed these two packages, enter the following lines of code in your command line:
pip install requests
pip install termcolor
After installing the packages, we can now import them into our python environment using the code below:
import requests
from termcolor import colored as clapi_key = 'YOUR API KEY'
We have now successfully imported the essential packages into our environment along with storing the API key into the api_key
variable.
2. Basic Program for Extracting Financial News
In this section, we are going to build a very simple Python programing to extract financial news, and the goal of this particular section is not to build the perfect program but to get some understanding of the financial news API provided by EOD Historical Data. The following code snippet is a basic program that will extract news according to the given input:
def get_stock_news(stock, api_key):
url = f'https://eodhistoricaldata.com/api/news?api_token={api_key}&s={stock}'
news_json = requests.get(url).json()news = []
for i in range(10):
title = news_json[-i]['title']
news.append(title)
print(cl('{}. '.format(i+1), attrs = ['bold']), '{}'.format(title))
return news
amzn_news = get_stock_news('AMZN', api_key)
In the above code, we are first defining a function named get_stock_news
that takes the symbol of the stock we want to get news about (symbol
), and the API key (api_key
) as parameters. Inside the function, we are creating the API URL based on the given inputs (stock’s symbol and API key) and storing it into the url
variable. Let’s now take some time to break down the structure of the API URL. Here is the URL:
https://eodhistoricaldata.com/api/news?api_token={api_key}&s={stock}
The first parameter of the above URL is the api_token
parameter where we specify the API key and the next parameter is s
which takes the symbol of the stock as input. These two are the primary or mandatory parameters but still, there are a lot of secondary parameters available for the purpose of tuning and customization.
Coming to the code, after storing the API URL, we are using the get
function provided by the Requests package to call the API, and finally, stored the API response in JSON format into the news_json
variable. The upcoming lines of code are pretty much about representing the extracted data in a meaningful way which is optional but tremendously helpful.
So, after extracting the financial news, we are creating a for-loop with the underlying idea of printing out the title of the last ten news about the stock and appending the titles to the news
list. Finally, we are returning the news
and called the created function to extract the ten latest news about Amazon and the output looks like this:
3. Advanced Program for Extracting Financial News
In the previous section, we aimed just to create a basic program but now let’s take this one step forward by building an advanced program to customize the API to our needs. When compared to the previous section, the code won’t differ much other than the change in the API URL. With that being said, the code would look something like this:
def get_customized_news(stock, start_date, end_date, n_news, api_key, offset = 0):
url = f'https://eodhistoricaldata.com/api/news?api_token={api_key}&s={stock}&limit={n_news}&offset={offset}&from={start_date}&to={end_date}'
news_json = requests.get(url).json()news = []
for i in range(len(news_json)):
title = news_json[-i]['title']
news.append(title)
print(cl('{}. '.format(i+1), attrs = ['bold']), '{}'.format(title))
return news
tsla_news = get_customized_news('TSLA', '2021-11-09', '2021-11-11', 15, api_key, 0)
Like I said before, the structure of the code is more or less similar to that of the previous section but here we are playing around with the other parameters of the API. In the above code, the function we defined has five primary parameters which are the stock’s symbol (symbol
), the starting date of the news feed (start_date
), the ending date of the news feed (end_date
), number of news we want as the output (n_news
), API key (api_key
), and one optional parameter (offset
).
Like how we inspected the API URL in the previous section, let’s do the same in this section too since we have made some modifications. Here is the URL:
https://eodhistoricaldata.com/api/news?api_token={api_key}&s={stock}&limit={n_news}&offset={offset}&from={start_date}&to={end_date}
The first two parameters of the URL are the same as we saw before which are the api_token
, and the s
parameter. Then we have the limit
parameter where we have to mention the length of the output (minimum being 1, and the maximum is 1000). Followed by that, we have the from
and to
parameters whose names are self-explanatory. In between exists the offset
parameter whose default value is 0 but can be changed if needed.
Going back to the code, we are doing all the same stuff we did before to represent the extracted data using a for-loop, and finally, we are returning the values and calling the created function to get a customized news feed of Tesla. The output of the code is a list that looks like this:
In this article, we have learned everything we need to know about the EOD Historical Data’s financial news API and how can it be used to create basic programs as well as customized advanced programs which extract news according to our needs in Python.
Let’s now talk about areas of improvement. This article should only be considered as a glimpse of the applications of the EOD Historical Data’s financial news API and can be taken to the next level in several different ways. One such interesting way is to build a Sentiment Analysis application that will analyze the news feed extracted using the API and reveal its state whether good, bad, or neutral. People can use this application to note the current trend of the stock and make their investment decisions accordingly.
To conclude, the news is one of the greatest influencers of the market and the one who holds a strong edge of it can only succeed among the rest. It is agreeable that it was not possible for people to gain access to real-time news for an enormous amount of stocks in the past but thanks to resources like the financial news API provided by EOD Historical Data, anyone from anywhere is capable of doing it now. With that being said, you’ve arrived at the end of the article and hope you learned something new and useful from it.