Let’s take a look at the components of a Node.js application before we make a “Hello, World!” application. The three key components of a Node.js application are as follows:
- Import necessary modules To load Node.js modules, we utilise the require directive.
- Create a server, similar to Apache HTTP Server, that will listen for client requests.
- Read the HTTP request made by the client, which can be a browser or a console, and return the response The server built in the previous step will read the HTTP request made by the client, which may be a browser or a console, and return the response.
Step 1: Creating a Node.js application is to import the required modules.
To load the http module, we use the need directive and save the resulting HTTP instance into a http variable as follows:
require("http"); var http = require("http");
Step 2: Make a Server
We use the newly formed http instance to invoke the http.createServer() function to create a server instance, which we then bind to port 8081 using the server instance’s listen method. Pass it a function with the request and response arguments. Create an example implementation that returns “Hello World” every time.
http.createServer(function (request, response) { // Send the HTTP header // HTTP Status: 200 : OK // Content Type: text/plain response.writeHead(200, {'Content-Type': 'text/plain'}); // Send the response body as "Hello World" response.end('Hello World\n'); }).listen(8081); // Console will print the message console.log('Server running at http://127.0.0.1:8081/');
Step 3: Verify the Request and Response
Let’s combine steps 1 and 2 in a file named main.js and start our HTTP server like this:
var http = require("http"); http.createServer(function (request, response) { // Send the HTTP header // HTTP Status: 200 : OK // Content Type: text/plain response.writeHead(200, {'Content-Type': 'text/plain'}); // Send the response body as "Hello World" response.end('Hello World\n'); }).listen(8081); // Console will print the message console.log('Server running at http://127.0.0.1:8081/');
To start the server, run the main.js script as follows:
main.js $ node
Check the output. The server has begun to run.
Server running at http://127.0.0.1:8081/
Make a Request to the Node.js Server
Open http://127.0.0.1:8081/ in any browser and observe the following result.
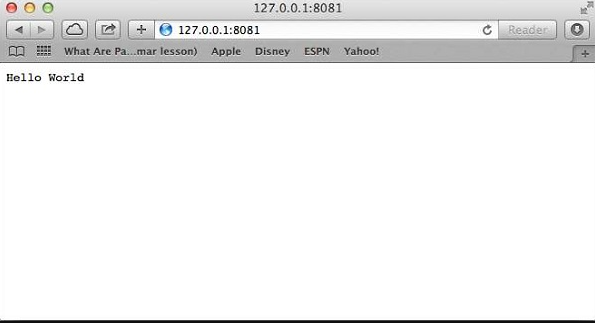
Congratulations, your first HTTP server is up and running, and it’s listening on port 8081 for all HTTP requests.