Java is object oriented programming OOP language so it provide the feature of Java Inheritance. Inheritance is the process of inheriting some or all feature of a class by another class. Inheritance provides a hierarchal structure to the code. Class features can be variables and methods. Inheritance works between classes. The classes in Java inheritance are:
- superclass (parent class)
- subclass (child class)
Superclass
The concept of superclass is same as base or parent class in C++ programming language. The class from which another class inherits few or all features, is superclass. However, the superclass cannot contain features of its subclasses. A superclass can have multiple subclasses.
Subclass
The concept of subclass is same as derived or child class in C++ programming language. The class that inherits few or all features of other class is subclass. A subclass can have only one superclass therefore, Java does not support multiple inheritance through classes.
extends Keyword
The extend keyword is used to create the relationship of inheritance between a superclass and a subclass. The syntax for creating inheritance is:
Here, the name of superclass is SuperClass and the name of subclass is SubClass. The extend keyword followed by superclass name is written after the name of subclass to define inheritance.
Following example shows the demonstration of Java Inheritance: The code below creates a superclass Calculator. It has two methods add() and substract(). The subclass CalculatorExtended is the subclass that extends from the superclass Calculator. It inherits all the variables (z) and methods (add() and subtract()) from its superclass. The subclass has its own method multiply().
The objects of subclass CalculatorExtended have access to all three methods its own and the superclass’ too. But the objects of superclass Calculator have access two only the two methods (add() and subtract()) that are defined in its scope.
class Calculator { int c; public void add(int a, int b) { c = a + b; System.out.println("The result of addition of the given numbers is : "+c); } public void subtract(int a, int b) { c = a - b; System.out.println("The result of subtraction of the given numbers is : "+c); } } public class CalculatorExtended extends Calculator { public void multiply(int a, int b) { c = a * b; System.out.println("The result of product of the given numbers is : "+c); } public static void main(String args[]) { int i = 10, j = 15; CalculatorExtended demo = new CalculatorExtended(); demo.add(i, j); demo.subtract(i, j); demo.multiply(i, j); } }
In the above program, when an object of CalculatorExtended subclass is created, a copy of all methods and fields of the superclass create memory in this object. Therefore, by using the objects of the subclass we can also access the members of a superclass. The output of the above code after compilation is:
Relation between Superclass and Subclass
The subclass to superclass relationship is a is-a relationship. For instance, consider the following examples:
- Fiction is a Book
- Car is a Vehicle
- Circle is a Shape
- Part-time employee is an Employee
and the list goes on. Here, Fiction, Car, Circle and Part-time employee are considered as subclasses, while Book, Vehicle, Shape and Employee are considered to be superclasses.
Types of Java Inheritance
There are following basic types of Java Inheritance
- Single Inheritance
- Multi level Inheritance
- Hierarchal Inheritance
Single Inheritance
So far we have read about Single Inheritance through above examples. Single Inheritance is the inheritance where only one class inherits from one superclass. The structure of Single Inheritance is shown in the figure below:

The below example shows another program that uses single inheritance:
class A { public void print_greetings() { System.out.print("Welcome to "); } } class B extends A { public void print_website() { System.out.println("TutorialsArt"); } } public class Main { public static void main(String[] args) { B obj = new B(); obj.print_greetings(); obj.print_website(); } }
The output of the above code after compilation is :
Multilevel Inheritance
The Multilevel Inheritance is the inheritance in which a subclass inherits members from another subclass. It creates a relationship of grand parents and grand children. The structure of Multilevel Inheritance is shown in the figure below:
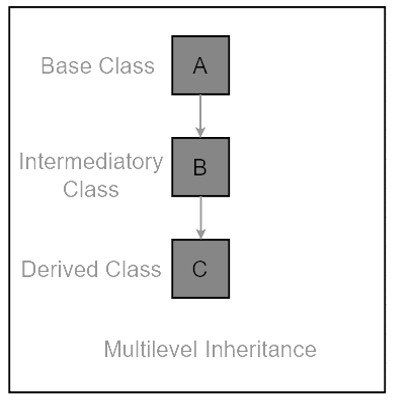
Here, class C inherits from class B and class B inherits from class A. Which make class A the grandparent class. The class B is both parent and child class hence it is intermediate class. It is both superclass and subclass. Whereas, the class C is only a subclass.
The below example demonstrates the concept of Multilevel Inheritance:
class A { public void print_greetings() { System.out.print("Welcome to "); } } class B extends A { public void print_website() { System.out.print("TutorialsArt"); } } class C extends B { public void print_language() { System.out.print(", Java Language"); } } public class Main { public static void main(String[] args) { C obj = new C(); obj.print_greetings(); obj.print_website(); obj.print_language(); } }
The output of the above code after compilation is:
Hierarchal Inheritance
Hierarchal Inheritance is the Java inheritance in which a superclass has more than one subclasses. All subclasses inherit all the members of superclass. The subclasses can have their own members too that will not be accessible to other subclasses. The structure of Hierarchal Inheritance is shown in the figure below:

Here, subclasses B, C and D inherit from superclass A. The below example demonstrates the concept of hierarchal inheritance:
class A { public void print_greetings() { System.out.print("Welcome to "); } } class B extends A { public void print_website() { System.out.println("TutorialsArt"); } } class C extends A { public void print_website() { System.out.println(" Google"); } } class D extends A { public void print_website() { System.out.println(" Youtube"); } } public class Main { public static void main(String[] args) { B obj1 = new B(); obj1.print_greetings(); obj1.print_website(); C obj2 = new C(); obj2.print_greetings(); obj2.print_website(); D obj3 = new D(); obj3.print_greetings(); obj3.print_website(); } }
The output of the above code after compilation is :
final Class
The final keyword is used to create a final class. Final class cannot have any subclasses and hence it stops Java inheritance. If user wants to restrict to create subclass from a class then it should be made final case. If user tries to create subclass from a final class then in this compiler will give an error. The syntax for defining a final class is:
No class can be inherited from the final class ClassName.