A C++ Library Management Project with straightforward and simple code. Essentially, we will program various components to add, erase, or update books. There are a lot more components we will use in the library the executives framework project. How about we have a concise gander at the components of this LMS project.
Features of C++ Library Management Project
Students Details-We will actually want to add or refresh the understudy subtleties like name, roll number, and so on These subtleties will be put away in various factors.
Add/Remove/Update Books-This library the board framework task will be customized to add, eliminate, or update books effectively that will make it more proficient.
Complete Books Details-This C++ Library Management Project will remember every one of the total subtleties of books present for the store.
Verification You can undoubtedly login to the LMS administration with various jobs. Assuming you are an administrator, you will get the administrator id and secret phrase from which you can without much of a stretch access the administrator administrations and the equivalent for every one of the clients.
Data set All the data added or eliminated will be refreshed in the data set. On the off chance that you are an administrator, you can get to and alter the information base
C++ Library Management Project Explanation:
Adding Books
0. Exit
1.Add new Book
2. Display All Books
3. Search Books
4. Dispose off Books
5. Modify Details
6. List of Disposed Books
Search Books by
- ID
- Title
- Category
- Author
- Price Range
//**************************************************************************************************************// // This C++ Library Management Project has been written by TutorialsArt.com // Distribution of the code is permitted if and only if you do not delete these lines. //**************************************************************************************************************// //*************************************************************** // HEADER FILES //**************************************************************** #include<fstream.h> #include<conio.h> #include<stdio.h> #include<process.h> #include<string.h> #include<iomanip.h> //*************************************************************** // Book Class //**************************************************************** class Cls_Book { char Book_No[6]; char Book_Name[50]; char Author_Name[20]; public: void create_book() { cout<<"\nNEW BOOK ENTRY...\n"; cout<<"\nEnter The Cls_Book no."; cin>>Book_No; cout<<"\n\nEnter The Name of The Book "; gets(Book_Name); cout<<"\n\nEnter The Author's Name "; gets(Author_Name); cout<<"\n\n\nBook Created.."; } void Show_Book_details() { cout<<"\nBook no. : "<<Book_No; cout<<"\nBook Name : "; puts(Book_Name); cout<<"Author Name : "; puts(Author_Name); } void Modify_Book_details() { cout<<"\nBook no. : "<<Book_No; cout<<"\nModify Book Name : "; gets(Book_Name); cout<<"\nModify Author's Name of Book : "; gets(Author_Name); } char* Return_Book_No() { return Book_No; } void Show_report() {cout<<Book_No<<setw(30)<<Book_Name<<setw(30)<<Author_Name<<endl;} }; //class ends here class Cls_Student { char Registration_No[6]; char St_Name[20]; char St_B_no[6]; int token; public: void create_student() { clrscr(); cout<<"\nNEW STUDENT ENTRY...\n"; cout<<"\nEnter The Registration no. "; cin>>Registration_No; cout<<"\n\nEnter The Name of The Student "; gets(St_Name); token=0; St_B_no[0]='/0'; cout<<"\n\nStudent Record Created.."; } void show_student_details() { cout<<"\\nRegistration no. : "<<Registration_No; cout<<"\nStudent Name : "; puts(St_Name); cout<<"\nNo of Book issued : "<<token; if(token==1) cout<<"\nBook No "<<St_B_no; } void Modify_Student_Details() { cout<<"\\nRegistration no. : "<<Registration_No; cout<<"\nModify Student Name : "; gets(St_Name); } char* Ret_Admission_no() { return Registration_No; } char* Ret_st_b_no() { return St_B_no; } int Ret_token() { return token; } void Add_Token() {token=1;} void Reset_Token() {token=0;} void getstbno(char t[]) { strcpy(St_B_no,t); } void Show_report() {cout<<"\t"<<Registration_No<<setw(20)<<St_Name<<setw(10)<<token<<endl;} }; //class ends here //*************************************************************** // global declaration for stream object, object //**************************************************************** fstream fp,fp1; Cls_Book bk; Cls_Student st; //*************************************************************** // function to write in file //**************************************************************** void Write_Book_details() { char ch; fp.open("Cls_Book.dat",ios::out|ios::app); do { clrscr(); bk.create_book(); fp.write((char*)&bk,sizeof(Cls_Book)); cout<<"\n\nDo you want to add more record..(y/n?)"; cin>>ch; }while(ch=='y'||ch=='Y'); fp.close(); } void Write_Student_Details() { char ch; fp.open("Cls_Student.dat",ios::out|ios::app); do { st.create_student(); fp.write((char*)&st,sizeof(Cls_Student)); cout<<"\n\ndo you want to add more record..(y/n?)"; cin>>ch; }while(ch=='y'||ch=='Y'); fp.close(); } //*************************************************************** // function to read specific record from file //**************************************************************** void Search_Book(char n[]) { cout<<"\nBOOK DETAILS\n"; int flag=0; fp.open("Cls_Book.dat",ios::in); while(fp.read((char*)&bk,sizeof(Cls_Book))) { if(strcmpi(bk.Return_Book_No(),n)==0) { bk.Show_Book_details(); flag=1; } } fp.close(); if(flag==0) cout<<"\n\nBook does not exist"; getch(); } void Search_Student(char n[]) { cout<<"\nSTUDENT DETAILS\n"; int flag=0; fp.open("Cls_Student.dat",ios::in); while(fp.read((char*)&st,sizeof(Cls_Student))) { if((strcmpi(st.Ret_Admission_no(),n)==0)) { st.show_student_details(); flag=1; } } fp.close(); if(flag==0) cout<<"\n\nStudent does not exist"; getch(); } //*************************************************************** // function to modify record of file //**************************************************************** void Modify_Book_details() { char n[6]; int found=0; clrscr(); cout<<"\n\n\tMODIFY BOOK REOCORD.... "; cout<<"\n\n\tEnter The Cls_Book no. of The Cls_Book"; cin>>n; fp.open("Cls_Book.dat",ios::in|ios::out); while(fp.read((char*)&bk,sizeof(Cls_Book)) && found==0) { if(strcmpi(bk.Return_Book_No(),n)==0) { bk.Show_Book_details(); cout<<"\nEnter The New Details of Cls_Book"<<endl; bk.Modify_Book_details(); int pos=-1*sizeof(bk); fp.seekp(pos,ios::cur); fp.write((char*)&bk,sizeof(Cls_Book)); cout<<"\n\n\t Record Updated"; found=1; } } fp.close(); if(found==0) cout<<"\n\n Record Not Found "; getch(); } void Modify_Student_Details() { char n[6]; int found=0; clrscr(); cout<<"\n\n\tMODIFY STUDENT RECORD... "; cout<<"\n\n\tEnter The admission no. of The Cls_Student"; cin>>n; fp.open("Cls_Student.dat",ios::in|ios::out); while(fp.read((char*)&st,sizeof(Cls_Student)) && found==0) { if(strcmpi(st.Ret_Admission_no(),n)==0) { st.show_student_details(); cout<<"\nEnter The New Details of Cls_Student"<<endl; st.Modify_Student_Details(); int pos=-1*sizeof(st); fp.seekp(pos,ios::cur); fp.write((char*)&st,sizeof(Cls_Student)); cout<<"\n\n\t Record Updated"; found=1; } } fp.close(); if(found==0) cout<<"\n\n Record Not Found "; getch(); } //*************************************************************** // function to delete record of file //**************************************************************** void Delete_Student_Details() { char n[6]; int flag=0; clrscr(); cout<<"\n\n\n\tDELETE STUDENT..."; cout<<"\n\nEnter The admission no. of the Student You Want To Delete : "; cin>>n; fp.open("Cls_Student.dat",ios::in|ios::out); fstream fp2; fp2.open("Temp.dat",ios::out); fp.seekg(0,ios::beg); while(fp.read((char*)&st,sizeof(Cls_Student))) { if(strcmpi(st.Ret_Admission_no(),n)!=0) fp2.write((char*)&st,sizeof(Cls_Student)); else flag=1; } fp2.close(); fp.close(); remove("Cls_Student.dat"); rename("Temp.dat","Cls_Student.dat"); if(flag==1) cout<<"\n\n\tRecord Deleted .."; else cout<<"\n\nRecord not found"; getch(); } void Delete_Book_Details() { char n[6]; clrscr(); cout<<"\n\n\n\tDELETE BOOK ..."; cout<<"\n\nEnter The Book no. of the Book You Want To Delete : "; cin>>n; fp.open("Cls_Book.dat",ios::in|ios::out); fstream fp2; fp2.open("Temp.dat",ios::out); fp.seekg(0,ios::beg); while(fp.read((char*)&bk,sizeof(Cls_Book))) { if(strcmpi(bk.Return_Book_No(),n)!=0) { fp2.write((char*)&bk,sizeof(Cls_Book)); } } fp2.close(); fp.close(); remove("Cls_Book.dat"); rename("Temp.dat","Cls_Book.dat"); cout<<"\n\n\tRecord Deleted .."; getch(); } //*************************************************************** // function to display all students list //**************************************************************** void Display_All_Students() { clrscr(); fp.open("Cls_Student.dat",ios::in); if(!fp) { cout<<"ERROR!!! FILE COULD NOT BE OPEN "; getch(); return; } cout<<"\n\n\t\tSTUDENT LIST\n\n"; cout<<"==================================================================\n"; cout<<"\tAdmission No."<<setw(10)<<"Name"<<setw(20)<<"Book Issued\n"; cout<<"==================================================================\n"; while(fp.read((char*)&st,sizeof(Cls_Student))) { st.Show_report(); } fp.close(); getch(); } //*************************************************************** // function to display Books list //**************************************************************** void Display_All_Books() { clrscr(); fp.open("Cls_Book.dat",ios::in); if(!fp) { cout<<"ERROR!!! FILE COULD NOT BE OPEN "; getch(); return; } cout<<"\n\n\t\tBook LIST\n\n"; cout<<"=========================================================================\n"; cout<<"Book Number"<<setw(20)<<"Book Name"<<setw(25)<<"Author\n"; cout<<"=========================================================================\n"; while(fp.read((char*)&bk,sizeof(Cls_Book))) { bk.Show_report(); } fp.close(); getch(); } //*************************************************************** // function to issue Cls_Book //**************************************************************** void Issue_Book() { char sn[6],bn[6]; int found=0,flag=0; clrscr(); cout<<"\n\nBOOK ISSUE ..."; cout<<"\n\n\tEnter The Cls_Student's admission no."; cin>>sn; fp.open("Cls_Student.dat",ios::in|ios::out); fp1.open("Cls_Book.dat",ios::in|ios::out); while(fp.read((char*)&st,sizeof(Cls_Student)) && found==0) { if(strcmpi(st.Ret_Admission_no(),sn)==0) { found=1; if(st.Ret_token()==0) { cout<<"\n\n\tEnter the Cls_Book no. "; cin>>bn; while(fp1.read((char*)&bk,sizeof(Cls_Book))&& flag==0) { if(strcmpi(bk.Return_Book_No(),bn)==0) { bk.Show_Book_details(); flag=1; st.Add_Token(); st.getstbno(bk.Return_Book_No()); int pos=-1*sizeof(st); fp.seekp(pos,ios::cur); fp.write((char*)&st,sizeof(Cls_Student)); cout<<"\n\n\t Book issued successfully\n\nPlease Note: Write current date in backside of Cls_Book and submit within 15 days fine Rs. 1 for each day after 15 days period"; } } if(flag==0) cout<<"Book no does not exist"; } else cout<<"You have not returned the last Cls_Book "; } } if(found==0) cout<<"Student record not exist..."; getch(); fp.close(); fp1.close(); } //*************************************************************** // function to deposit Cls_Book //**************************************************************** void Deposit_Book() { char sn[6],bn[6]; int found=0,flag=0,day,fine; clrscr(); cout<<"\n\nBOOK DEPOSIT ..."; cout<<"\n\n\tEnter The student’s admission no."; cin>>sn; fp.open("Cls_Student.dat",ios::in|ios::out); fp1.open("Cls_Book.dat",ios::in|ios::out); while(fp.read((char*)&st,sizeof(Cls_Student)) && found==0) { if(strcmpi(st.Ret_Admission_no(),sn)==0) { found=1; if(st.Ret_token()==1) { while(fp1.read((char*)&bk,sizeof(Cls_Book))&& flag==0) { if(strcmpi(bk.Return_Book_No(),st.Ret_st_b_no())==0) { bk.Show_Book_details(); flag=1; cout<<"\n\nBook deposited in no. of days"; cin>>day; if(day>15) { fine=(day-15)*1; cout<<"\n\nFine has to deposited Rs. "<<fine; } st.Reset_Token(); int pos=-1*sizeof(st); fp.seekp(pos,ios::cur); fp.write((char*)&st,sizeof(Cls_Student)); cout<<"\n\n\t Book deposited successfully"; } } if(flag==0) cout<<"Book no does not exist"; } else cout<<"No Cls_Book is issued..please check!!"; } } if(found==0) cout<<"Student record not exist..."; getch(); fp.close(); fp1.close(); } //*************************************************************** // INTRODUCTION FUNCTION //**************************************************************** void Library_Intro() { clrscr(); gotoxy(35,11); cout<<"LIBRARY"; gotoxy(35,14); cout<<"MANAGEMENT"; gotoxy(35,17); cout<<"SYSTEM"; cout<<"\n\nMADE BY : YOUR NAME"; cout<<"\n\nSCHOOL : SCHOOL NAME"; getch(); } //*************************************************************** // ADMINISTRATOR MENU FUNCTION //**************************************************************** void Menu_For_Admin() { clrscr(); int ch2; cout<<"\n\n\n\tADMINISTRATOR MENU"; cout<<"\n\n\t1.CREATE STUDENT RECORD"; cout<<"\n\n\t2.DISPLAY ALL STUDENTS RECORD"; cout<<"\n\n\t3.DISPLAY SPECIFIC STUDENT RECORD "; cout<<"\n\n\t4.MODIFY STUDENT RECORD"; cout<<"\n\n\t5.DELETE STUDENT RECORD"; cout<<"\n\n\t6.CREATE BOOK "; cout<<"\n\n\t7.DISPLAY ALL BOOKS "; cout<<"\n\n\t8.DISPLAY SPECIFIC BOOK "; cout<<"\n\n\t9.MODIFY BOOK "; cout<<"\n\n\t10.DELETE BOOK "; cout<<"\n\n\t11.BACK TO MAIN MENU"; cout<<"\n\n\tPlease Enter Your Choice (1-11) "; cin>>ch2; switch(ch2) { case 1: clrscr(); Write_Student_Details();break; case 2: Display_All_Students();break; case 3: char num[6]; clrscr(); cout<<"\n\n\tPlease Enter The Admission No. "; cin>>num; Search_Student(num); break; case 4: Modify_Student_Details();break; case 5: Delete_Student_Details();break; case 6: clrscr(); Write_Book_details();break; case 7: Display_All_Books();break; case 8: { char num[6]; clrscr(); cout<<"\n\n\tPlease Enter The Cls_Book No. "; cin>>num; Search_Book(num); break; } case 9: Modify_Book_details();break; case 10: Delete_Book_Details();break; case 11: return; default:cout<<"\a"; } Menu_For_Admin(); } //*************************************************************** // THE MAIN FUNCTION OF PROGRAM //**************************************************************** void main() { char ch; Library_Intro(); do { clrscr(); cout<<"\n\n\n\tMAIN MENU"; cout<<"\n\n\t01. BOOK ISSUE"; cout<<"\n\n\t02. BOOK DEPOSIT"; cout<<"\n\n\t03. ADMINISTRATOR MENU"; cout<<"\n\n\t04. EXIT"; cout<<"\n\n\tPlease Select Your Option (1-4) "; ch=getche(); switch(ch) { case '1':clrscr(); Issue_Book(); break; case '2':Deposit_Book(); break; case '3':Menu_For_Admin(); break; case '4':exit(0); default :cout<<"\a"; } }while(ch!='4'); } //*************************************************************** // END OF PROJECT //***************************************************************
C++ Library Management Project Image
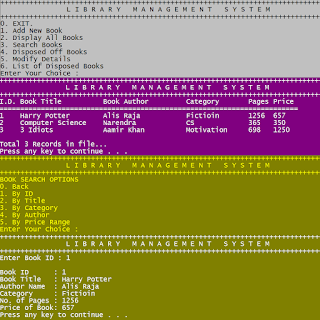