C++ Strings are of two types:
- C++ String class
- C-Style Character String
C++ String class
Strings is one of the most common data types used in C++ programming language. String type variables store characters in double quotes. They are objects of string class that is part of standard C++ library. In order to use string variables, the common practice is to include header file in the code. The syntax for adding C++ Strings header file is:
The syntax for initializating a string variable is:
For example, the code to initialize name variable is given below:
Program can also take input from user in the form of strings. The following code takes string input from user and displays it.
#include <iostream> #include <string> using namespace std; int main() { string name; cout << "What is your name? "; cin >> name; cout << "Greetings, " << name << "!\n"; return 0; }
The output of above code after compilation is:
In order to enter an entire sentence as an input, getline function is used. The getline function takes two arguments. The first argument is the keyword cin and the second argument is string variable. The syntax of getline function is:
The code below shows the use of getline function to get sentences from user as string input.
#include <iostream> #include <string> using namespace std; int main () { string fname; cout << "Please enter your full name " << endl; getline (cin, fname); cout << "Hello " << fname << ", Welcome to TutorialsArt!" << endl; return 0; }
The output of above code after compilation is:
C-Style Character String
C-Style character string is actually an array of character type that contains characters in double quotes. It is a single-dimension array that ends with a null character ‘\0’. The length of this array is one greater than the total number of characters. The below image shows the Character array and indices having value “Hello”.
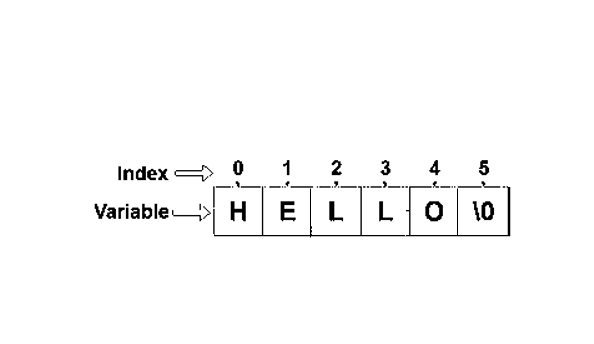
The syntax for initializing character string is:
A programmer can define strings in more than one way. The second syntax for initializing string array is:
The third syntax for initializing array is:
For example in the code below, there are three character arrays initialized in different ways having same outputs.
#include <iostream> #include <string> using namespace std; int main () { char str1[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; char str2[] = {'H', 'e', 'l', 'l', 'o', '\0'}; char str3[6] = "Hello"; cout << str1 << endl; cout << str2 << endl; cout << str3 << endl; return 0; }
The output of above code after compilation is:
Note that all three statements give the same output.
String Manipulations
C++ programming language provides various operations on strings and C-style character arrays. There are multiple functions that serve the same purpose. Programmer can choose any of them. These built in functions and their purposes are listed below:
- strlen(str1); returns the length of C-style character string str1.
- str1.size(); returns size of the string str1.
- str1.length(); returns size of the string str1.
- str1.resize(n); resizes the size of string str1 to a new size n that is an integer.
- str1.capacity(); returns the capacity of string str1 that may be equal to or grater than its size.
- strcpy(str1, str2); It is C-style character string function that copies the contents present in string str2 into string str1. C++ also allows the programmer to copy the contents of one string to another using the assignment = operator.
- str1.swap(str2); swaps the contents of two strings str1 and str2.
- strcat(str1, str2); It is C-style character string function that concatenates the value present in string str2 to the end of the string str1. C++ also allows the programmer to concatenate two strings using the + operator.
- str1.append(str2); appends the string str2 to the end of the string str1.
- strcmp(str1, str2); compares two strings str1 and str2 and returns integer value based on the comparison. If str1 is equal to str2 it returns 0. If str1>str2, it returns integer value greater than 0. If str2>str1 it returns integer value less than 0.
- strchr(str1, ch); checks for the first occurrence of the character ch in the string str1 and returns a pointer to that occurrence.
- strstr(str1, str2); checks for the first occurrence of the string str2 in the string str1 and returns a pointer to that occurrence.
- getline(cin, str): takes two arguments cin and a string variable. It takes stream of text from user as input and omits terminators like spaces.
- str1.push_back(ch); adds character ch to the end of string str1.
- str1.pop_back(); deletes the last character from the string str1.
- str.begin(); returns an iterator pointing to the start of string str1.
- str.end(); returns an iterator pointing to the last element of string str1.
The code in the example below shows two strings s1 and s2, how to concatenate them and how to calculate the size of resulting string s3 in two different ways.
#include <iostream> #include <string> using namespace std; int main () { string s1 = "C++"; string s2 = "Tutorials"; string s3; int size, len; s3 = s1+s2; cout << "The result of concatenation of s1 and s2 is " << s3 << endl; size = s3.size(); len = s3.length(); cout << "The size of string s3 is " << size << endl; cout << "The length of string s3 is " << len << endl; return 0; }
The output of above code after compilation is:
Programmer can achieve same functionality using C-style character strings and their operations. Below code shows string manipulations on C-style character strings s1 and s2.
#include <iostream> #include <cstring> using namespace std; int main () { char s1[10] = "C++"; char s2[10] = "Tutorials"; int len ; strcat(s1, s2); cout << "The result of concatenation of s1 and s2 is: " << s1 << endl; len = strlen(s1); cout << "The length of concatenated string s1 is: " << len << endl; return 0; }
The output of the above code after compilation is:
The code in below example uses push_back() and pop_back() functions to add and remove character from a string s1. Here the program adds and removes full stop (.) to and from the end of the string s1.
#include<iostream> #include<string> using namespace std; int main() { string s1 = "Tutorials"; cout << "The original string is :"; cout << s1 << endl; s1.push_back('.'); cout << "The string after push_back operation is :"; cout << s1 << endl; s1.pop_back(); cout << "The string after pop_back operation is :"; cout << s1 << endl; return 0; }
The output of the above code after compilation is: