C++ Multidimensional arrays are arrays within an array. Multidimensional arrays can be two-dimensional (2D), three-dimensional (3D), and so on. The syntax for writing multidimensional arrays is:
Here ArrayType is the data type of array. ArrayName is the name of array. ArraySize is the size of array. Size of array should be greater than 0. For example, a two dimensional array is declared as:
A three dimensional array is declared as:
Multidimensional array form a matrix or table having multiple rows and columns.
Size of multidimensional arrays
The size of multidimensional array is equal the product of sizes of all dimensions. The number of elements present in multidimensional array is same as its size. For example, the size of two-dimensional array int arr2[5][7] is (5×7) = 35. And the size of three-dimensional array int arr3[3][5][7] is (3x5x7) = 105. That means array arr2 can contain 35 elements and array arr3 can contain 105 elements.
C++ Two-dimensional Array Initialization
The syntax for initializing two-dimensional array is similar to single arrays. There are two methods of initializing two-dimensional arrays. The first method, the nested braces method contains a set of single arrays in another curly brace. Below example shows how to initialize an array of 3 rows and 5 columns using nested curly braces:
The size of first array determines the number of rows and the size of second array determines the number of columns of two-dimensional array.
The second method to initialize two-dimensional arrays is simple. It is similar to single array initialization. Below example shows how to initialize a two-dimensional array of 3 by 5:
The purpose of both syntaxes is same.
C++ Three-dimensional Array Initialization
The syntax for initializing three-dimensional array is similar to two-dimensional arrays. Just like two-dimensional arrays, three-dimensional arrays also have two syntaxes for initialization. One method is nested braces method. The example below shows to initialize three-dimensional array int arr3[2][3][4]:
The size of first array determines the number of sets. In the above case its 2, so there are two sets of arrays. The size of second array determines the number of arrays in each set. In the above example its 3, so there are three arrays in each set. The size of third arrays determines the number elements in each array of each set. Here it is 4, so there are four elements in each array. The size of the three-dimensional array given above is 2x3x4 =24 which is also the number of total elements of the array.
The other method of initializing three-dimensional array is:
Accessing Array Elements
The method to access 2D and 3D array elements is similar. 2D array elements have two integers in square brackets while 3D array elements have 3 integers in 3 square brackets.
Two-dimensional Arrays
Each element of a two-dimensional array contains two coordinates. These two coordinates give the right address of the respective element. For example, an array having 3 rows and 3 columns is shown below:

Following example shows to access element at row 0 and column 1:
Three-dimensional Arrays
Each element of a three-dimensional array contains three coordinates. These three coordinates give the right address of the respective element. For example, a 3D array x[3][3][3] is shown below:
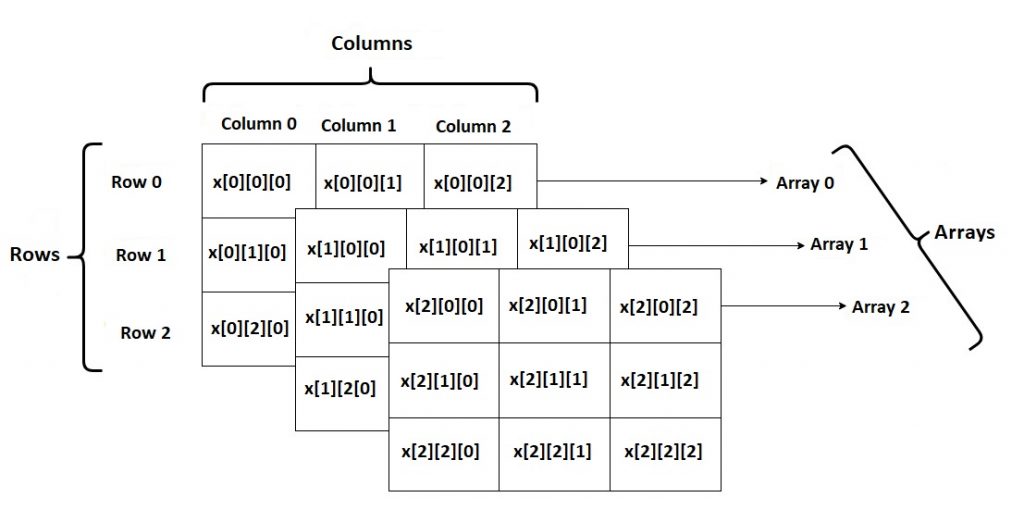
Following example shows to access element at row 0, column 1, array 2:
Printing Arrays
Programmers can print 2D and 3D arrays using two and three loops respectively. The below example shows how to print all elements of a 2D array:
#include<iostream> using namespace std; int main() { int arr[3][5] = {{0,1,2,3,4}, {5,6,7,8,9}, {10,11,12,13,14}}; for (int i = 0; i < 3; i++) { for (int j = 0; j < 5; j++) { cout << "Element at arr[" << i << "][" << j << "]: "; cout << arr[i][j]<<endl; } } return 0; }
The output of the above code is:
Similarly, three-dimensional arrays take three loops to print all the elements. The below example shows how to print all elements of a three-dimensional array.
#include<iostream> using namespace std; int main() { int arr[3][3][3] = { { {0,1,2}, {3,4,5}, {6,7,8} }, { {9,10,11}, {12,13,14}, {15,16,17} } , { {18,19,20}, {21,22,23}, {24,25,26} }, }; cout << "Elements of 3D array are: {"; for (int i = 0; i < 3; ++i) { for (int j = 0; j < 3; ++j) { for (int k = 0; k < 3; ++k) { cout << arr[i][j][k] << ", "; } } } cout << "}"; return 0; }