Loops
Quite often there comes a situation when a programmer needs to repeat a piece of code multiple times.
Why is Repetition Needed?
Repetition provides following purposes:
- Duplication
- Taking input, sum, and average of multiple numbers using a limited number of variables
For example, to add five numbers we may have two different algorithms:
- Declare a variable for each number, input the numbers and add the variables together
- Variable that contains the sum of the numbers
In first case we have to use five variables and then add them up while in second case we just need to take one variable and create a loop which will run five times and we will get more efficient program.
In this scenario, the best practice is to avoid writing the same lines of code again and again. To handle this situation, C++ programming language has loops.
C++ Loops
C++ Programming language provides various control structures that allow for more complicated execution paths. A loop statement allows us to execute a statement or group of statements multiple times. For instance, if a programmer needs to print a sentence 10 times then instead of writing cout statement 10 times, the programmer can use loops.
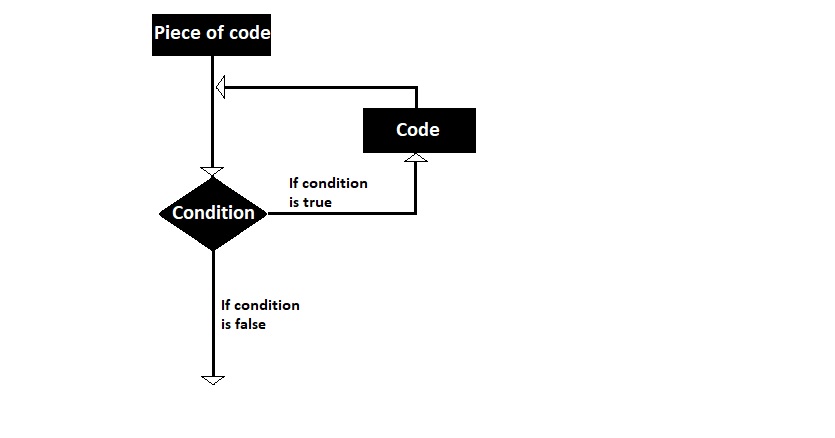
There are four types of loops in C++ programming language:
- while loop
- for loop
- do…for loop
- Nested Loop
While Loop
The general form of the while statement is:
while is a reserved word or keyword.
- Code can be simple or compound statements
- Condition acts as a decision-maker and is usually a logical expression
- The program executes the code if the condition evaluates to true then reevaluates the condition and keeps executing the code within the loop until the condition is no longer true. When the condition becomes false, the program exits the loop.
For example, the below code displays even numbers between 1 to 21 using while loop.
#include <iostream> using namespace std; int main() { int num = 1; while (num <= 21) { if (num % 2 == 0){ cout << num<< " ";} num++; } return 0; }
The above code gives the following result after compilation:
Infinite While Loop
The while loop that continues to execute endlessly and never stops is the infinite while loop. It includes statements in the loop body that assure exit condition will always be false.
For example, below code shows the logic for an infinite while loop:
#include <iostream> using namespace std; int main() { int x=1; while(x<=6) { cout<<"Value of variable is: "<<x<<endl; x--; } return 0; }
The output of the above code is infinite. It keeps executing and printing current values of variable ‘x’ until the code execution is stopped.
Another syntax for writing Infinite While Loop is:
In the above example, the condition is While loop is always true, hence the loops run for infinite times until the memory is fully utilized.
Counter-Controlled While Loop
Counter-Controlled while loop takes a variable, often named as index or counter that keeps the count of loops. When the index reaches a certain value (the loop bound) the loop will end. Count–controlled repetition is definite repetition because the number of repetitions is known before the loop begins executing.
Count-controlled repetition requires the following:
- control variable (or loop counter)
- initial value of the control variable
- increment (or decrement) by which the control variable is modified each iteration through the loop
- condition that tests for the final value of the control variable
The below code takes ten values from user as input, adds them, and displays the sum using while loop. The variable ‘i’ is the loop counter. Since the size of array is 10 so the loop will be executed 10 times. The initial value of the loop counter is 0. In the body of while loop, the loop counter increases by 1 after it satisfies the condition being smaller than 10.
#include <iostream> using namespace std; int main() { int sum = 0; int arr[10]; int i = 0; cout << "Enter 10 integers:" << endl; while (i<10) { cin >> arr[i]; sum = sum + arr[i]; i++; } cout << "The sum of 10 integers entered is: " << sum; return 0; }
The output of the above code is:
Sentinel-Controlled While Loop
Sentinel Controlled Loop is the loop where the programmer doesn’t know exactly how many times loop body will execute. For this reason, it is also called as indefinite repetition loop. It takes a special variable called Sentinel variable that controls the flow of loop. The condition tests the sentinel variable value and the loop ends when sentinel is encountered.
For example, in the code below, the loops keeps asking the user to enter a number until he/she enters 5.
#include <iostream> using namespace std; int main() { int i; cout << "Enter your lucky number between 1 to 10" << endl; cin >> i; while (i!=5) { cout << "Try again" << endl; cin >> i; } cout << "Congratulations!"; return 0; }
The output of above code depends on the input by user. The program will end as soon as user enters 5, else it will keep asking user for input.
Flag-Controlled While Loop
Flag controlled While loop has a bool variable. The bool variable is defined and initialized to serve as a flag. The while loop continues until the flag variable value flips (true becomes false or false becomes true). A flag-controlled while loop uses a bool variable to control the loop
In the following example, the program asks the user to enter numbers from the keyboard which are then added together. To stop the addition the user enters a negative number. The while loop keeps adding the numbers together unless user enters a negative number. When user enters a negative number, it sets the flag variable false and hence programs ends.
#include <iostream> using namespace std; int main() { double sum = 0.0; double value; bool nonNegative = true; while(nonNegative) { cout << "Enter a value" << endl; cin >> value; if(value < 0) { nonNegative = false; cout << "Program ended as you entered a negative number" << endl; } else { sum+=value; cout << "Sum of input numbers is " << sum << endl; } } return 0; }
The output of the above code after compilation is:
For Loop
A for loop has three control statements. The general form of the for statement is:
The initial statement, loop condition, and update statement are called for loop control statements. For is a reserved word in C++ programming language.
The for loop executes in the following sequence:
- The compiler executes the initial statement
- The compiler validates the loop condition
- If the loop condition is true, the compiler executes the block of code inside the curly braces
- Then the compiler executes the update statement
- The compiler repeats steps 2,3,4 until the loop condition evaluates to false.
When the loop condition is false, the compiler exists the loop. The initial statement usually initializes a variable (called the for loop control, or for indexed, variable).
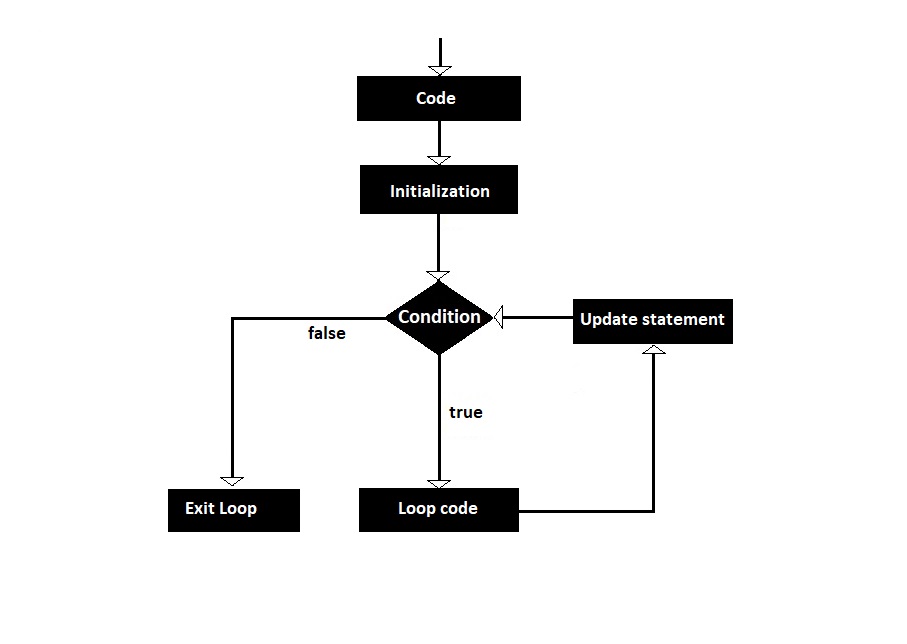
The simple code below uses for loop to print the first 10 positive integers. The first statement (int i = 0) initializes and declares a variable. Loop will run for the value of the variable. The second statement (i < 10) determines how many times the loop will run. The third statement increases the value of variable i by 1 until it reaches the value 10. Here, i is smaller than 10 and i starts from 0 so the loop will run 10 times.
#include <iostream> using namespace std; int main() { for (int i = 0; i < 10; i++) { cout << i << endl; } return 0; }
The above code gives the following output:
Here is another example that shows various update operations other than increment of 1. There are two for loops in the code below.
#include <iostream> using namespace std; int main() { for (int j = 5; j > 0; j--) { cout << j << " "; } for (int j = 0; j < 15; j += 2) { cout << j << " "; } return 0; }
The output of the above code after compilation is:
The first for loop initializes the value of j to 5 and evaluates the condition j > 0 (checks that value of j is greater than 0) and then executes the body of loop. When the first time loop’s body executes, it is called the first iteration. It displays the value of j (which is 5) and then updates the statement (decrements the value of j by 1). In the second iteration, it displays a new value of j that is 4. Then again it evaluates the loop condition (checks the value of j is greater than 0). Then it executes the loop body and displays the value of j (j=3). This is repeated until the value of j becomes equal to 0. The final result of the first loop will be 5 4 3 2 1. In this case the for loop iterates 6 times. On the sixth iteration, compiler exits the for loop as it no longer validates the loop condition (j > 0).
The second for loop initializes the value of j to 0 and then evaluates the loop condition (checks if the value of j is less than 15). After that compiler executes the body of loop. Here it displays the value of j which is 0 in the first iteration. And then executes the update statement (increments the value of j by 2). Now the value of j is 2. The loop again evaluates the loop condition (checks the value of j is less than 15) and executes the loop body and displays the value of j that is 2 in the second iteration. Compiler repeats these steps until the value of j becomes equal to 15. And end result will be 0 2 4 6 8 10 12 14.
Infinite For Loop
An Infinite For loop never terminates and keeps running forver. The infinite for loop omits all three statements—initial statement, loop condition, and update statement. The following is the syntax of infinite for loop:
For example, the below code prints Hello World unlimited number of times:
#include <iostream> using namespace std; int main() { for (; ;) { cout << "Hello World" << endl; } return 0; }
The output of the above code after compilation is:
The code keeps printing “Hello World” endlessly unless the code is stopped.
Do…While Loop
Do…While loop works similar to the while loop. The difference between both loops is the order of execution. In Do While loop the compiler first executes the body of the loop and then checks the loop condition. Do…while loop has an exit condition and always iterates at least once unlike for and while loops. The flowchart below helps explain the working of Do…While loop.
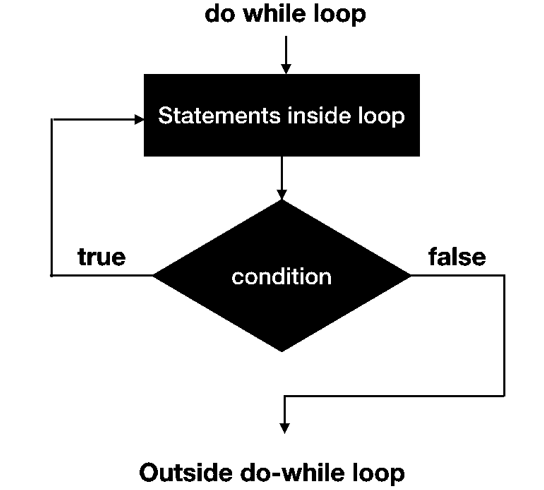
The syntax of Do…While Loop is:
The code below prints numbers from 1 to 10 using Do…While loop. There are total 11 iterations. In 11th iteration, the compiler exists the loop.
#include <iostream> using namespace std; int main() { int n = 1; do { cout << n << " "; ++n; } while (n < 11); return 0; }
The output of the above code after compilation is:
Infinite Do…While Loop
Do…While loop continues to execute infinitely if while condition never becomes false. The syntax for writing infinite while loop is:
In the above example, the while condition is always true so the block of code inside curly braces of do will execute infinitely.
Nested Loops
In C++ programming language, nested loops are loops within loops. Programmer can add up to 256 levels of nested loops. It may be for, while, or do…while loop. The syntax for writing nested loop statements using for loops is:
In the same way, the syntax for writing nested loops using while loops is:
Similarly, the syntax for writing nested loops using Do…While loop is:
For example, in the code below, nested for loops can print tree patterns using asterisk. In the first line, programmer wants to print one star, in the second line two stars and so on.
#include <iostream> using namespace std; int main() { int lines = 5; for(int i = 1; i <= lines; ++i) { for(int star = 1; star <= i; ++star) { cout << "* "; } cout << "\n"; } return 0; }
The output of above code after compilation is:
Since the program prints 5 lines so in the first for loop, the condition is (i <= 5). It means the first for loop will execute for 5 times. The value of ‘i’ in the first iteration is 1, in the second iteration its value is 2, and so on.
The value of variable ‘j’ within second loop controls the number of asterisks in each line. The number of asterisks in each line is equal to the number of line. There is one asterisk in one line, 2 in second line, 3 in third and so on. So the number of times second for loop iterates is equal to line number.
Comments are closed.