In Python, Tkinter widgets allow access to specific GUI-geometry management methods. These geometry management methods used to organize widgets throughout the parent widget area. Widgets can be arranged by determining the size and position of components. The geometry managers always set the positioning and sizing. There are 3 main GUI-Geometry Management given below:
- The pack( ) Geometry Manager
- The grid( ) Geometry Manager
- The place( ) Geometry Manager
The pack( ) Geometry Manager:
The pack( ) Geometry Manager uses a packing algorithm in GUI-Geometry Management. This manager is used to place widgets in Frame in a specific order. The packing algorithm has two initial steps, 1st it Computes a rectangular area known as a parcel that holds the widget and with blank space, it fills the remaining width in the window. 2nd is to Center the widget in the parcel unless a different location is specified.
Syntax:
Options in pack() method:
Options | Description |
side | This option analyze which side of the parent widget packs against Top, Bottom, Left, or Right. |
expand | Due to this option, the widget expands to fill any space when set to true. |
fill | This option determines the extra space widget’s allocated by the packer or remain in None (default), X (fill only horizontally), Y (fill only vertically), or Both (fill both horizontally and vertically). |
Example:
from tkinter import * appWindow = Tk() appWindow.title("Window Title-Tutorialsart.com") appWindow.geometry('350x200') frame = Frame(appWindow) frame.pack() bottomframe = Frame(appWindow) bottomframe.pack( side = BOTTOM ) greenbutton = Button(frame, text="Green Foreground", fg="green") greenbutton.pack( side = LEFT ) blackbutton = Button(bottomframe, text="Black Foreground", fg="black") blackbutton.pack( side = BOTTOM) appWindow.mainloop()

The grid( ) Geometry Manager:
The grid( ) geometry manager is the most flexible of the GUI-Geometry Management. In the parent widget, the grid( ) manager organizes widgets in a 2-dimensional table-like structure. In this 2-D table, each cell in the resulting table can hold a widget. And the master widget is split into a number of rows and columns.
Syntax:
Parameters in grid() method:
Parameters | Description |
rowspan | Show the number of row widgets occupies. By default is1. |
columnspan | Show the number of columns widget occupies. By default is 1. |
column | The column to put the widget in. By default is 0. |
row | The row to put the widget in. By default, the first row is still empty. |
padx, pady | Show the number of pixels to the pad widget outside the widget’s borders. |
ipadx, ipady | Show the number of pixels to the pad widget inside the widget’s borders. |
sticky | By default, with sticky=”, the widget is centered in its cell. sticky can be the string concatenation of zero or compass directions. |
Example:
from tkinter import * import tkinter appWindow = Tk() appWindow.title("Window Title-Tutorialsart.com") appWindow.geometry('350x200') for r in range(2): for c in range(3): tkinter.Label(appWindow, text='R%s/C%s'%(r,c), borderwidth=2 ).grid(row=r,column=c) appWindow.mainloop()
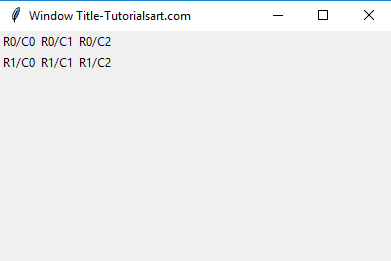
The place( ) Geometry Manager:
The Place ( ) geometry manager is the simplest manager of Tkinter GUI-Geometry Management. It organizes widgets by placing them in a specific position in the parent widget. It allows you explicitly set the position and size of a window. It can’t be used for ordinary window and dialog layouts.
Syntax:
Parameters in place() method:
Parameters | Description |
x, y | Shows the horizontal and vertical offset in pixels. |
height, width | Shows the height and width in pixels. |
relheight, relwidth | Shows the height and width as a float between 0.0 and 1.0. |
bordermode | Shows the INSIDE to indicate that other options refer to the parents inside, OUTSIDE otherwise. |
relx, rely | Shows the horizontal and vertical offset as a float between 0.0 and 1. |
anchor | Shows the exact spot of widget like compass directions indicating the corners and sides of the widget. |
Example:
from tkinter import * import tkinter appWindow = Tk() appWindow.title("Window Title-Tutorialsart.com") appWindow.geometry('350x200') # button widget b1 = Button(appWindow, text="ADD") b1.place(relx=1, x=-2, y=2, anchor=NE) # label widget l = Label(appWindow, text="Label") l.place(anchor=NW) # button widget b2 = Button(appWindow, text="PLACE()") b2.place(relx=0.5, rely=0.5, anchor=CENTER) appWindow.mainloop()
