To take Python User Input, we use input() function in Python Programming Language, it asks for an input from the user and returns a string value, no matter what value you have entered, all values will be considered as strings values.
.png)
Almost all interesting Python programs accept input from the user at some point. You can start accepting user input in your programs by using the input() function in Python. The input function displays a message to the user describing the kind of input you are looking for, and then it waits for the user to enter a value.
input() Syntax:
Parameters: prompt: (Optional) A string, representing the default message before taking input.
Return Value: A string.
The following example takes the user input using the input() method.
name = input("Enter your name: ") print("Hello", name + "!")
Above, the input()
function takes the user’s input in the next single line, so whatever user writes in a single line would be assign to to a variable name
. So, the value of a name
would be whatever user has typed and printed using the print function.
User input in Python 2.7
In Python 2 we can read input from standard input device i.e. the keyboard using the following two built-in functions. But as we all know the official support to Python 2 is ended. So it’s better to learn Python 3 now.
raw_input
input
The example of taking the user input using raw_input is as follows
username = raw_input("Enter username:") print("Username is: " + username)
Execution flow
As you play around with this program you’ll notice that it actually stops it’s flow of execution when awaiting input from the user. In pervious programs we’re written they seem to execute and be done in a matter of milliseconds. But in this case if you wait 10 minutes before you decide to input something, the computer will wait along with you.
This is because when the computer see’s that it’s supposed to accept input it stops everything it’s doing and waits. In a lot of cases, the input that the computer receives from the user is vital to it being able to perform it’s task, whatever that may be.
Many programs you’ll encounter (and eventually write) will rely on the user to tell them what to do and when it’s okay for them to stop. Once we discover more advanced topics we’ll eventually be able to write programs that have menus and give the user an option of what they want to do! But this is where it all starts
Python user input and EOFError Example:
EOFError in python is one of the exceptions to handle errors and is raised in scenarios such as the interruption of the input() function in both python version 2.7 and python version 3.6, as well as other versions after version 3.6 or when the input() function reaches the unexpected end of the file in python version 2.7, i.e. the functions do not read any data before the end of the input is encountered.
Methods such as read() must return a string that is empty at the end of the file, which is inherited in Python from the exception class that is inherited in BaseException class. This mistake often happens with online IDEs.
If the input() function did not read any data then the python code will throw an exception i.e. EOFError.
and the output gives and error pretend in the below image.
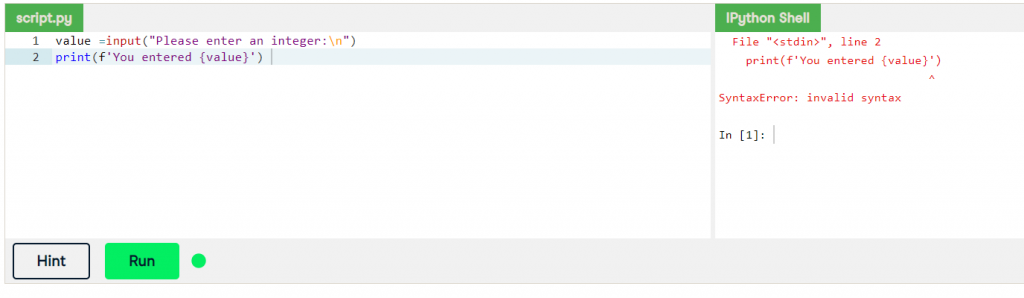