A String is a collection of characters. They are formed by a list of characters. Python strings are used to display text. To access elements of the string, Square brackets are used. The Python string is a sequence of Unicode characters.
As we know the computer does not deal with the characters, it only deals with binary numbers. When we enter any character on the computer, first it was converted into binary numbers. This conversion of characters to numbers is called Encoding. And converting those numbers again into characters to show us on the screen this process called Decoding. For this conversation the most popular encodings ASCII and Unicode encodings used.
Creating strings:
To create strings, we can use single , double or even triple quotes.
As “python” and ‘python’ are same. And to print string, print ( ) function is used.
#Creating a String with single Quotes String = 'John is a good programmer' print("String with Single Quotes: ") print(String)
Accessing characters in strings:
To access characters in strings most commonly used method is by Index. Each character has a unique index through which, the character can be access easily. Index starts from 0 and can end on any number. The index should be an integer, not a float or any other type, as it will raise an error, named Type Error. If an entered character is out of index range then, there will be an error, known as Index Error.
Example given below shows the index system,
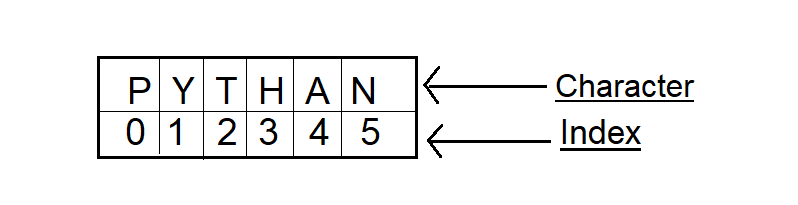
#Accessing string characters with index in Python string = "python" print('string = ', string) #first character print('string[0] = ', string[0]) #third character print('string[2] = ', string[2])
Updating/Deleting Strings:
Python programming is unchangeable, it means if you have entered any value or character once in any string you can’t change or delete that character. But we can simply reassign different strings to the same name. updating or deleting a single character is not possible there will be an error, but updating and deleting the entire string can be posible.
For Updating entire string:
#Updating entire String in python String1 = "Jam learned python" print("First String: ") print(String1) # Updating a String String1 = "Jam is a good programmer" print("\nUpdated String: ") print(String1)
For deletion of entire string:
Deletion of comp;ete string is possible with the use keyword del. But if, we try to print the string, this will display an error because String is deleted and is unavailable to be shown or print.
#Deletion of entire String in python String1 = "John is not a good programmer" print("First String: ") print(String1) # Deleting a String using del keyword del String1 print("\nDeleting entire String: ") print(String1)
Escape Characters:
Some characters can not be used in strings, because they are reserved to use in your code, they known as Escaped Characters. In a programming language, the “backslash (\)” character is known as an escape character. It has a special meaning when used inside the string. The escapes character escapes the characters in a string for a brief moment to introduce unique inclusion. It could be indicating a new line or printing double-quotes.
Escape Sequence is the sequence of characters after a backslash. In Python, there are different escape sequences that have a unique meaning.
The full table for escaped characters in python is as given below,
Escape Sequence | Description |
\newline | Backslash and newline ignored |
\’ | Single quote |
\” | Double quote |
\a | ASCII Bell |
\b | ASCII Backspace |
\f | ASCII Formfeed |
\n | ASCII Linefeed |
\r | ASCII Carriage Return |
\t | ASCII Horizontal Tab |
\v | ASCII Vertical Tab |
\\ | Backslash |
\ooo | A character with octal value ooo |
\xHH | A character with hexadecimal value HH |
Python String Operators:
In this section, some operators are applied on strings. some operators are given below,
Operators | Description |
+ | Concatenation – Adds values on the other side of the operator. |
* | Repetition – creates new strings, concatenating multiple copies of the same string. |
[ ] | Slice – Gives the character from the index. |
[ : ] | Range Slice – Gives the characters from the range. |
% | Format – Performs String formatting. |
in | Membership – Returns true if a character exists in the string. |
not in | Membership – Returns true if a character does not exist in the string. |
r/R | Raw String |
To understand above mentioned operators, let consider example given below,
# Python String Operations string1 = 'Tom' string2 ='programmer' #applying concatenation + print('string1 + string2 = ', string1 + string2) #applying repetition * print('string1 * 3 =', string1 * 3) #applying slice [] print('string2[5]=', string2[5]) #applying range slice [:] print('string1[1:2]',string1[1:2] ) #applying in/ not in >> 'o' in 'Tom' >> 'e' not in 'programmer'
String Formatting Operator:
In python, % this operator is known as string format operator. The method of formatting string using % operator is same as that of ‘printf’ in C programming language.
#formatting string print "Tom is a %s!" % ('programmer') print "John got %d marks!" % ('89')
Following is the list of symbols which can be used with %.
Operator | Conversion |
%c | Character |
%s | String conversion via str() |
%i | Signed decimal integer |
%d | Signed decimal integer |
%u | Unsigned decimal integer |
%o | Octal integer |
%G | The shorter of %f and %E |
%g | The shorter of %f and %e |
%e | Exponential notation (with lowercase ‘e’) |
%x | Hexadecimal integer (lowercase letters) |
%X | Hexadecimal integer (UPPERcase letters) |
%f | Floating-point real number |
%E | Exponential notation (with UPPERcase ‘E’) |
There are also some supported symbols present in it. some of them are given below with their functionality description.
Symbol | Functionality |
* | It specifies width or precision. |
+ | Display the sign |
– | Left justification |
<sp> | To leave a blank space before a positive number |
# | To Add the octal / hexadecimal leading ‘0x’ or ‘0X’, depending on whether ‘x’ or ‘X’ were used. |
0 | Pad from left with zeros (instead of spaces) |
% | ‘%%’ leaves you with a single literal ‘%’ |
(var) | Mapping variable (dictionary arguments) |
m.n. | m is the minimum total width and n is the number of digits to display after the decimal point (if appl.) |
Unicode String:
In Python, strings are stored internally as 8-bit ASCII, while Unicode strings stored as 16-bit Unicode. This have some characters set. In the program, u simple is used to show the Unicode string.
Example:
Output:
Built-in String Methods:
Python have some built-in methods that can be used on strings in the programe. the table given below show some built-in methods.
Methods | Description |
capitalize() | Converts the first character to upper case. |
casefold() | Converts string into lower case. |
center() | Returns a centered string. |
count() | Returns the number of times a specified value occurs in a string. |
encode() | Returns an encoded version of the string. |
endswith() | Returns true if the string ends with the specified value. |
expandtabs() | Sets the tab size of the string. |
find() | Searches the string for a specified value and returns the position of where it was found. |
format() | Formats specified values in a string. |
format_map() | Formats specified values in a string. |
index() | Searches the string for a specified value and returns the position of where it was found. |
isalmun() | Returns True if all characters in the string are alphanumeric. |
isalpha() | Returns True if all characters in the string are in the alphabet. |
isdecimal() | Returns True if all characters in the string are decimals. |
isdigit() | Returns True if all characters in the string are digits. |
isidentifier() | Returns True if the string is an identifier. |
islower() | Returns True if all characters in the string are lower case. |
isnumeric() | Returns True if all characters in the string are numeric. |
isprintable() | Returns True if all characters in the string are printable. |
isspace() | Returns True if all characters in the string are whitespaces. |
istitle() | Returns True if the string follows the rules of a title. |
isupper() | Returns True if all characters in the string are upper case. |
join() | Joins the elements of an iterable to the end of the string. |
!just() | Returns a left justified version of the string. |
lower() | Converts a string into lower case. |
Istrip() | Returns a left trim version of the string. |
maketrans() | Returns a translation table to be used in translations. |
partition() | Returns a tuple where the string is parted into three parts. |
replace() | Returns a string where a specified value is replaced with a specified value. |
rjust() | Returns a right-justified version of the string. |
rpartition() | Returns a tuple where the string is parted into three parts. |
rsplit() | Splits the string at the specified separator, and returns a list. |
rfind() | Searches the string for a specified value and returns the last position of where it was found. |
rindex() | Searches the string for a specified value and returns the last position of where it was found. |
rstrip() | Returns a right trim version of the string. |
startswith() | Returns true if the string starts with the specified value. |
splitlines() | Splits the string at line breaks and returns a list. |
upper() | Converts a string into upper case. |
split() | Splits the string at the specified separator, and returns a list. |
translate() | Returns a translated string. |
strip() | Returns a trimmed version of the string. |
zfill() | Fills the string with a specified number of 0 values at the beginning. |
swapcase() | In swaps cases, the lower case becomes the upper case and vice versa. |
title() | Converts the first character of each word to upper case. |