Scripts in HTML pages cause the page to become unresponsive until the script is completely executed, which effects the performance of the page.
HTML Web Worker is fast and easy way for web content to run the scripts in the background. Tasks can be performed by the web worker threads without interfering with the user interface.
Web Worker run independent of other scripts, and it has no impact on page performance. While the HTML web worker is running in the background, you can continue to do whatever you want, such as: clicking, selecting etc.
HTML Web Worker Example
- HTML Main File:
<!DOCTYPE html> <html> <body> <p>Count numbers: <output id="result"></output></p> <button onclick="startcounter()">Start Counter</button> <button onclick="stopcounter()">Stop Counter</button> <script> var w; function startcounter() { if(typeof(Worker) !== "undefined") { if(typeof(w) == "undefined") { w = new Worker("counter-worker.js"); } w.onmessage = function(event) { document.getElementById("result").innerHTML = event.data; }; } else { document.getElementById("result").innerHTML = "Sorry, your browser does not support Web Workers"; } } function stopcounter() { w.terminate(); w = undefined; } </script> </body> </html>
Worker file
var i = 0; function timedCount() { i = i + 1; postMessage(i); setTimeout("timedCount()",500); } timedCount();
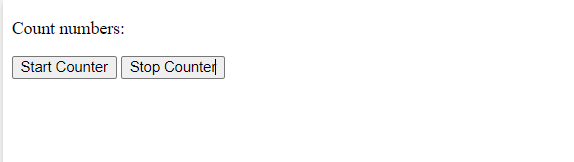
Web Worker Working
Now lets see how HTML Web Worker API can be operated.
Step 1: Check Browser Support:
Its better to check the browser support, before using web worker. Lets see the syntax for checking browser support.
if (typeof(Worker) !== "undefined") { // Some code..... } else { // Sorry! No Web Worker support.. }
Step 2: Create Web Worker File
Now create an external Web Worker javascript file. In the above example we created counter-worker.js file.
var i = 0; function timedCount() { i = i + 1; postMessage(i); setTimeout("timedCount()",500); } timedCount();
The postMessage() in the above file is used to send a message back to html page on which we are creating worker object.
Step 3: Create Worker
After checking browser support second step is to create worker in main html file using constructor and attach worker file.
w = new Worker("counter-worker.js");
Step 4:Recieve message using event listener
Now for receiving message from worker file create an event listener onmessage(). Just like below:
w.onmessage = function(event) { document.getElementById("result").innerHTML = event.data; }
When the worker file will post any message the code within event listener will be executed and the date will be stored in event.data.
Step 5:Terminate Worker
Use the terminate() method to end a web worker and free up browser/computer resources:
w.terminate();
The working of above example is explained in above steps so, that it become easy to understand code.
Web Worker and DOM
As Web Worker are in external files So, they do not have access to the following JavaScript objects:
- The parent object
- The document object
- The window object
Although Web Worker are in external files but can access the following objects:
- The location object
- The navigator object
- XMLHttpRequest can be accessed