JavaScript Array iteration methods operate on every array item. Following are some methods that are used for array iteration:
Array.filter() method
The filter()
the method is used to create a new array with array elements based on condition.
This example creates a new array from elements with a value larger than 20:
<!DOCTYPE html> <html> <body> <script> const n1 = [34, 13, 24, 5, 23, 245, 8]; const n2 = n1.filter(Fun); document.write(n2); function Fun(value, index, array) { return value > 20; } </script> </body> </html>
Output:

In the example above, the callback function does not use the index and array parameters, so they can be omitted:
<!DOCTYPE html> <html> <body> <script> const n1 = [34, 13, 24, 5, 23, 245, 8]; const n2 = n1.filter(Fun); document.write(n2); function Fun(value) { return value > 20; } </script> </body> </html>
Array.reduce() method
The reduce()
the method is used to run a function on each array element to produce a single value without affecting the original array. It works from left to right in the array.
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.reduce(Fun); document.write(sum); function Fun(total, value, index, array) { return total + value; } </script> </body> </html>
Output:

The example above does not use the index and array parameters. So, it can be omitted:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.reduce(Fun); document.write(sum); function Fun(total, value) { return total + value; } </script> </body> </html>
The reduce()
the method can accept starting value:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.reduce(Fun, 20); document.write(sum); function Fun(total, value, index, array) { return total + value; } </script> </body> </html>
Output:

Array.reduceRight() method
The reduceRight()
the method is used to run a function on each array element to produce a single value without affecting the original array. It works from right to left in the array.
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.reduceRight(Fun); document.write(sum); function Fun(total, value, index, array) { return total + value; } </script> </body> </html>
Output:

The example above does not use the index and array parameters. So, it can be omitted:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.reduceRight(Fun); document.write(sum); function Fun(total, value) { return total + value; } </script> </body> </html>
Array.forEach() method
The forEach()
the method is used to call a function once for each array element.
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let t = ""; n.forEach(Fun); document.write(t); function Fun(value, index, array) { t += value + "<br>"; } </script> </body> </html>
Output:
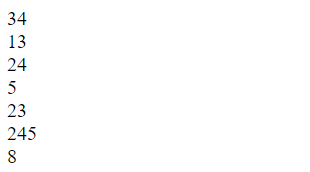
The example above uses only the value parameter. The example can be rewritten to:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let t = ""; n.forEach(Fun); document.write(t); function Fun(value) { t += value + "<br>"; } </script> </body> </html>
Array.map() method
The map()
method is used to create a new array by performing a function on each array element. It does not change the original array.
This example multiplies each array value by 3:
<!DOCTYPE html> <html> <body> <script> const n1 = [34, 13, 24, 5, 23, 245, 8]; const n2 = n1.map(Fun); document.write(n2); function Fun(value, index, array) { return value * 3; } </script> </body> </html>
Output:

When a callback function uses only the value parameter, the index and array parameters can be omitted:
<!DOCTYPE html> <html> <body> <script> const n1 = [34, 13, 24, 5, 23, 245, 8]; const n2 = n1.map(Fun); document.write(n2); function Fun(value) { return value * 3; } </script> </body> </html>
Array.every() method
The every()
the method is used to check if all array values pass a test. The following example will check if all array values are larger than 20:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.every(Fun); document.write(sum); function Fun(value, index, array) { return value > 20; } </script> </body> </html>
Output:

When a callback function uses the first parameter only (value), the other parameters can be omitted:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 245, 8]; let sum = n.every(Fun); document.write(sum); function Fun(value) { return value > 20; } </script> </body> </html>
Array.indexOf() method
The indexOf()
the method is used to search an array for an element value and return its position. The index starts from 0.
Syntax
array.indexOf(item, start)
Here:
- item: The item we need to search
- start: search starting pint
<!DOCTYPE html> <html> <body> <script> const language = ["Python", "C++", "C#", "Java"]; let i = language.indexOf("C++") + 1; document.write(i); </script> </body> </html>
Output:

If the item is not found the Array.indexOf()
returns -1.
If the item is available more than once, it will return the position of the first occurrence.
Array.lastIndexOf() method
Array.lastIndexOf()
work same as Array.indexOf()
, but it returns the position of the last occurrence of the specified element.
Syntax
array.lastIndexOf(item, start)
Here:
- item: The item we need to search
- start: search starting pint
<!DOCTYPE html> <html> <body> <script> const language = ["Python", "C++", "Python", "Java"]; let i = language.lastIndexOf("Python") + 1; document.write(i); </script> </body> </html>
Output:

Array.includes() method
The Array.includes()
is used to check if an element is present in an array.
Syntax
array.includes(search-item)
Array.find() method
The find()
the method is used to return the value of the first array element that passes a test function.
The below example will find the first element that is larger than 20:
<!DOCTYPE html> <html> <body> <script> const n = [34, 13, 24, 5, 23, 25, 8]; let f = n.find(Fun); document.write(f); function Fun(value, index, array) { return value > 20; } </script> </body> </html>
Output:
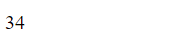
The function takes 3 arguments:
- The item value
- The item index
- The array itself
The Array.find() method
is not supported in older browsers.
Array.findIndex() method
The findIndex()
method is used to return the index of the first array element that passes a test function.
The below example will find the index of the first element that is larger than 20:
<!DOCTYPE html> <html> <body> <script> const n = [14, 13, 24, 5, 23, 25, 8]; let f = n.findIndex(Fun); document.write(f); function Fun(value, index, array) { return value > 20; } </script> </body> </html>
Output:

The function takes 3 arguments:
- The item value
- The item index
- The array itself
The Array.findIndex() method
is not supported in older browsers.
Array.from() method
The Array.from()
the method is used to return an Array object from any object with any iterable object or a length property.
Array.Keys() method
The Array.keys() method is used to return an Array Iterator object with the keys of an array.