The JavaScript break and continue statements are very important. The break statement is used to terminate or jump out of a loop and the continue statement is used to skip one iteration or jump over one iteration in the loop. Following are explanations of JavaScript Break and Continue with an example:
The Break Statement
The Break statement is used to terminate a loop or jump out of a loop.
<!DOCTYPE html> <html> <body> <script> for (let i = 1; i < 8; i++) { if (i === 6) { break; } document.write("Iteration # " + i + "<br>"); } </script> </body> </html>
Output:
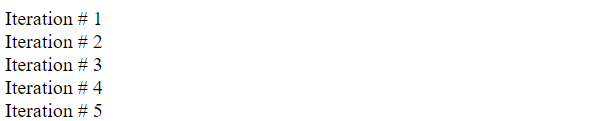
In the example above, the break
statement ends the loop when the loop counter is n == 6.
The Continue Statement
If a specified condition arrived in the code, the continue statement skips one iteration and may continue with the next iteration in the loop.
<!DOCTYPE html> <html> <body> <script> for (let i = 1; i < 12; i++) { if (i === 6) { continue; } document.write("Iteration # " + i + "<br>"); } </script> </body> </html>
Output:
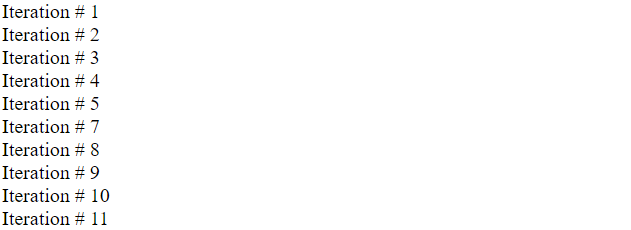
The above example skips the value of 6: