In the JavaScript Dates topic, you can learn how JavaScript supports date and you can create it. Date objects represent a single moment in time in a platform-independent format.
Time-zones
There are hundreds of time zones in our world. In JavaScript, we only care about two—Local Time and Coordinated Universal Time (UTC).
- Local time refers to the timezone your computer is in.
- UTC is synonymous with Greenwich Mean Time (GMT) in practice.
By default, almost every date method in JavaScript (except one) gives you a date/time in local time. You only get UTC if you specify UTC.
Creating JavaScript dates
We can create a date with new Date()
. There are possible ways to use new Date()
:
The date-String
In the date-string method, you create a date by passing a date-string into new Date
.
<!DOCTYPE html> <html> <body> <script> var d = new Date(); document.write(d); </script> </body> </html>
Output:

Creating JavaScript Dates with & without Arguments
There are seven arguments pass in the new Date() method. As you go left to right, you insert values in decreasing magnitude: year, month, day, hours, minutes, seconds, and milliseconds.
new Date()
Without arguments – create a Date
object for the current date and time:
<!DOCTYPE html> <html> <body> <script> let n = new Date(); alert(n); </script> </body> </html>
Output:
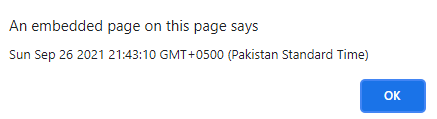
new Date(milliseconds)
Create a Date
object with the time equal to a number of milliseconds (1/1000 of a second) passed after the Jan 1st of 1970 UTC+0.
<!DOCTYPE html> <html> <body> <script> let Jan01_1970 = new Date(0); alert( Jan01_1970 ); let Jan02_1970 = new Date(24 * 3600 * 1000); alert( Jan02_1970 ); </script> </body> </html>
Output:
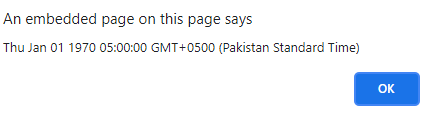
An integer number representing the number of milliseconds that have passed since the beginning of 1970 is called a timestamp.
It’s a lightweight numeric representation of a date. We can always create a date from a timestamp using new Date(timestamp)
and convert the existing Date
object to a timestamp using the date.getTime()
method (see below).
Dates before 01.01.1970 have negative timestamps, e.g.:
<!DOCTYPE html> <html> <body> <script> // 31 Dec 1969 let Dec31_1969 = new Date(-24 * 3600 * 1000); alert( Dec31_1969 ); </script> </body> </html>
Output:
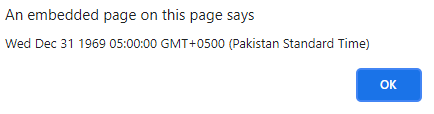
new Date(datestring)
If there is a single argument, and it’s a string, then it is parsed automatically. The algorithm is the same as Date.parse
uses, we’ll cover it later.
<!DOCTYPE html> <html> <body> <script> let date = new Date("2017-01-26"); alert(date); </script> </body> </html>
Output:
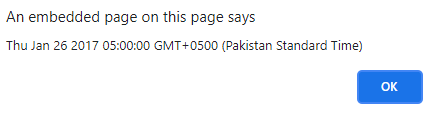
new Date(year, month, date, hours, minutes, seconds, ms)
Create the date with the given components in the local time zone. Only the first two arguments are obligatory.
- The
year
must have 4 digits:2013
is okay,98
is not. - The
month
count starts with0
(Jan), up to11
(Dec). - The
date
parameter is actually the day of month, if absent then1
is assumed. - If
hours/minutes/seconds/ms
is absent, they are assumed to be equal0
.
For instance:
The maximal precision is 1 ms (1/1000 sec):