In JavaScript, numbers are implemented in double-precision 64-bit binary format IEEE 754. Numbers are only one type, represent numbers with or without decimals.
JavaScript Numbers Exponentiation
The very large and very small JavaScript numbers can be written with exponent (e).
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var a = 324e3; var b = 233e-6; document.getElementById("tacode").innerHTML = a + "<br>" + b; </script> </body> </html>
Output:

JavaScript Numbers Format
Just like other programming languages, JavaScript does not define different types like integers, short, long, floating-point. It always follows IEEE 754 standard and represents a double-precision floating-point.
Its format stores data in 64 bit, where numbers stores in 0-51, exponent in 52-62 bits, and sign in bit 63.
Precision
- Integers show accuracy up to 15 digits.
- The maximum number of decimal 17 but floating point is not always 100% correct.
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var a = 0.2 + 0.1; document.getElementById("tacode").innerHTML = "0.2 + 0.1 = " + a; </script> </body> </html>
Output:

Adding Numbers and Strings
- If we add two numbers (10+9) the result will be number (19).
- If we add two strings (“10″+”9”) the result will be a string (109) concatenation.
- If we add a number and a string (10+”9″) the result will be a string (109) concatenation.
- If we add a string and a number (“10″+9) the result will also be a string (109) concatenation.
- JavaScript uses the + operator for both addition and concatenation.
- Numbers are added. Strings are concatenated.
- It you add two numbers and a string (10+5+ “9”) the result will be (159)
- The JavaScript interpret from left to right.
- First 10 + 5 is added because both are numbers.
- Then 15 + “9” is concatenated because z is a string.
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var a = 10; var b = 5; var c = "9"; var result = a + b + c; document.getElementById("tacode").innerHTML = result; </script> </body> </html>
Output:

Numeric Strings
JavaScript Numbers will work in all numeric operations with numeric strings. It will try to convert all strings to numbers in all numeric operations.
- If you subtract two strings (“100”-“10”) it will work and gives result 90.
- If you divide two strings ( “100”/ “10” ) it will also work and show result 10.
- If you multiply two strings ( “100” * “10) it will work and results 1000.
- But if you add two strings ( “100” + “10” ) it will not work.
Because JavaScript Numbers use + operator as concatenating the strings.
NaN- Not a Number:
In JavaScript numbers, Nan is a value that returns when you use a non-numeric value as a number for a numeric operation.
But if the string contains a numeric value the result will be a number as we learned above.
NaN is a number the typeof( ) Nan will returns its type “number”.
If you use NaN in a mathematical operation the result will also be Nan.
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var a = NaN; var b = 7; document.getElementById("tacode").innerHTML = a + b; </script> </body> </html>
Output:

If you use NaN with a string in a mathematical operation the result will concatenate the string and NaN.
<!DOCTYPE html> <html> <body> <p id="tacode"</p> <script> var a = NaN; var b = "7"; document.getElementById("tacode").innerHTML = a + b; // result will b NaN7 </script> </body> </html>
Output:

Infinity
In JavaScript Numbers, infinity is the number type value the typeOf() infinity will return the number. Infinity or negative infinity returns when you calculate a number outside the largest possible number.
Dividing a number by zero 0 also returns infinity.
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var Num = 3; var txt = ""; while (Num != Infinity) { Num = Num * Num; txt = txt + Num + "<br>"; } document.getElementById("tacode").innerHTML = txt; </script> </body> </html>
Output:
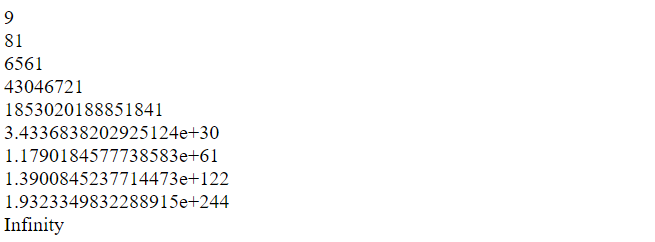
Hexadecimal
Hexadecimal number syntax uses a leading zero followed by a lowercase or uppercase Latin letter “X” (0x
or 0X
). “Identifier starts immediately after numeric literal”.
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var a = 0xAB; document.getElementById("tacode").innerHTML = "0xAB = " + a; </script> </body> </html>
Output:

- Never write a number with a leading zero (like 07).
- Some JavaScript versions interpret numbers as octal if they are written with a leading zero.
Numbers can be Objects:
Usually, numbers are created by literals but In JavaScript, Numbers can also be defined as objects. By using the keyword new such as var a = new Number(111); But there is no need to create numbers as objects.
<!DOCTYPE html> <html> <body> <p id="tacode"></p> <script> var a = 111; var b = new Number(111); document.getElementById("tacode").innerHTML = typeof a + "<br>" + typeof b; </script> </body> </html>
Output:

- Do not create Number objects. It slows down execution speed.
- The
new
keyword complicates the code. This can produce some unexpected results: