JavaScript loops are used to execute a block of code many times. Loops are very helpful if we want to run the same code, again and again, each time with a different value.
Instead of writing a print statement many times, we can apply a loop and execute it as many as we want. Because in case if we need to write a statement 100 times or more then it is very difficult to write so here loops can be used.
JavaScript Loops offer a quick and simple method for carrying out recurring activities. They promise to carry out iterations with a minimal amount of code. Iteration refers to how frequently you wish to repeat a job (that number can even be zero). Make sure you comprehend each one so you can use the appropriate loop statement in each case.
It example is given below:
Types of JavaScript Loops
Every programming language has two basic forms of loops, and JavaScript is no different. JavaScript Loops are also of two types which are:
Entry Controlled Loops: An entry controlled JavaScript Loops are loops in which the test condition is checked before entering the loop. The test condition in these loops determines whether or not the program will enter the loop. For instance, whereas, is among them.
Exit Controlled Loops: An exit controlled JavaScript Loops are loops in which the test condition is checked after the statements have been performed once. The test condition in these loops dictates whether or not the program will break the loop. Do…while loop is included in this category.
JavaScript loops support different kinds of loops:
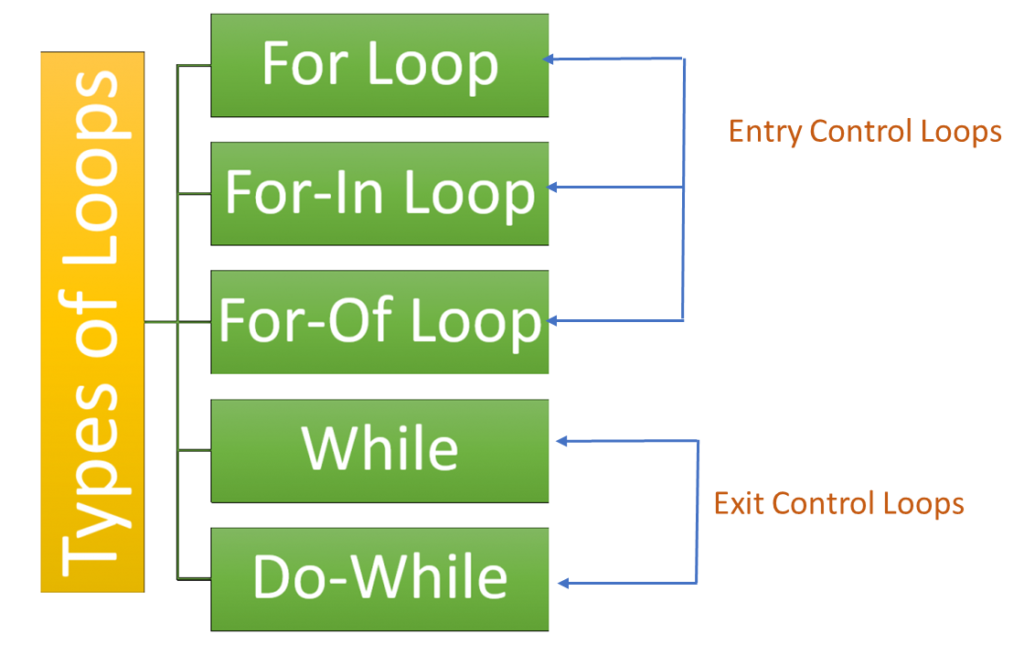
for
-It is used to loop a block of code a number of timesfor/in
– It is used to loop an object propertiesfor/of
– It is used to loop an iterable object valueswhile
– It is used to loop a block of code while a specified condition is truedo/while
– It is also used to loop a block of code while a specified condition is true
JavaScript for loop
JavaScript for loop is used to iterate a block of code a number of times until a condition is true. The syntax of JavaScript for loop is given below:
Here:
initialization: initialize the counter variable
condition: Here we define the condition that executes the code block.
increment: update the loop counter every time
The working of for loop is shown below using the Flowchart chart:
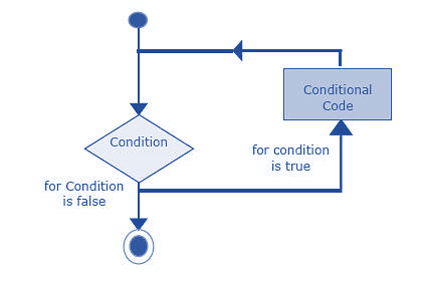
<!DOCTYPE html> <html> <body> <script> let a = ""; for (let n = 0; n < 8; n++) { document.write("Tutorials Art <br>"); } </script> </body> </html>
Output:
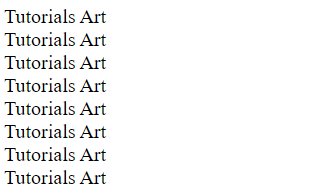
As per the above example, you can read:
- In the statement 1 variable before the loop starts (n=0).
- In the statement 2 defines the condition for loop to execute (n must be less than 8)
- In the statement 3 increase a value (n++) each time the code block in the loops has been executed.
JavaScript For Loop – Scope
Using var
in a loop:
Using let
in a loop:
As per the first example, we can redeclare the variable outside the loop using var. But as per the second example, we cannot redeclare the variable outside the loop using let.
JavaScript For In
To loops through the properties of an object or array JavaScript for in statement is used. Its syntax is given below:
Let’s understand it with basic example is given below:
<html> <body> <script> const student = {fn:"Ashi ", ln:"Ayaz", a:"25"}; for (var i in student) { document.write("<p>" + i + " = " + student[i] + "</p>"); } </script> </body> </html>
Output:
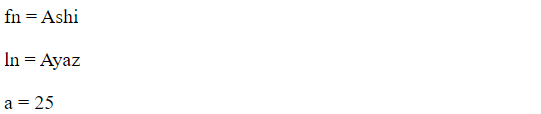
JavaScript For In Over Arrays
To loop over the properties of an Array we can use for in statement also:
Consider the following example to understand this concept:
<html> <body> <script> const n = [44, 42, 23, 87, 21]; for (var i in n) { document.write("<p>" + i + " = " + n[i] + "</p>"); } </script> </body> </html>
Output:
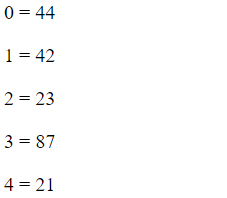
If the index order is important in the array then do not for in loop.
JavaScript For Of
avaScript for of statement used to iterate through the values of an iterate-able object. It is used to loop over iterate able data structures such as Strings, Maps, Arrays, NodeLists, and many others. Its syntax is given below:
Here:
variable – For every iteration, the value of the next property is assigned to the variable. Variable can be declared with const
, let
, or var
.
iterable – An object with iterable properties.
Following is an example to for of over an array:
<html> <body> <script> const n = [44, 42, 23, 87, 21]; for (var i of n) { document.write("<p>" + i + "</p>"); } </script> </body> </html>
Output:
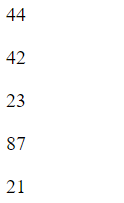
Following is an example of JavaScript for of over a string:
<html> <body> <script> const str = "Tutorials Art"; for (var i of str) { document.write("<p>" + i + "</p>"); } </script> </body> </html>
Output:
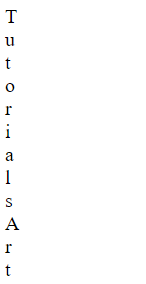
JavaScript While Loop
JavaScript While Loop is a very simple type of loop. Until a specific condition is true JavaScript while loop executes the block of code.
Syntax
Let’s understand this concept using the below example:
<html> <body> <script> var i = 0; while(i < 5) { document.write("<p>Iteration # " + i + "</p>"); i++; } </script> </body> </html>
Output:
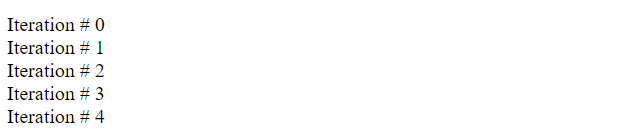
In case the increment of variable is not added the loop will continue and might crash the browser.
JavaScript Do While Loop
The do-while loop will execute the code block once, before checking if the condition comes true and repeat the loop until the condition comes true. Its syntax is given below:
Let’s understand this concept using the below example:
<html> <body> <script> var i = 0; do { document.write("<p>Iteration # " + i + "</p>"); i++; } while(i < 5); </script> </body> </html>
Output:
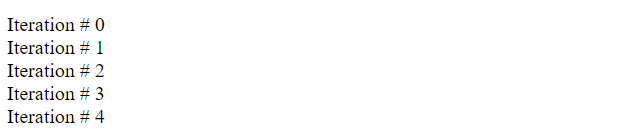
Comparing JavaScript while Loop and do-while Loop
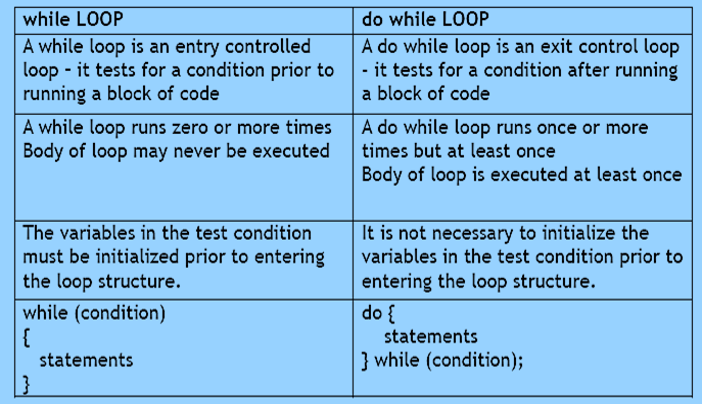