JavaScript Conditional statements are used to control some actions based on various conditions. For different decisions, we want to perform different actions. The JavaScript conditional statements can be used in code for this purpose. If the condition is true it performed an action or if the condition is false it performed another action.
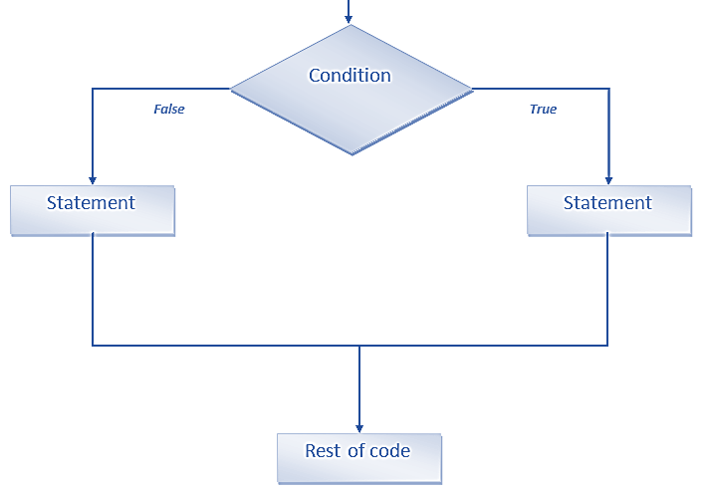
JavaScript Conditional Statements – Types
Following conditional statements are available in JavaScript.
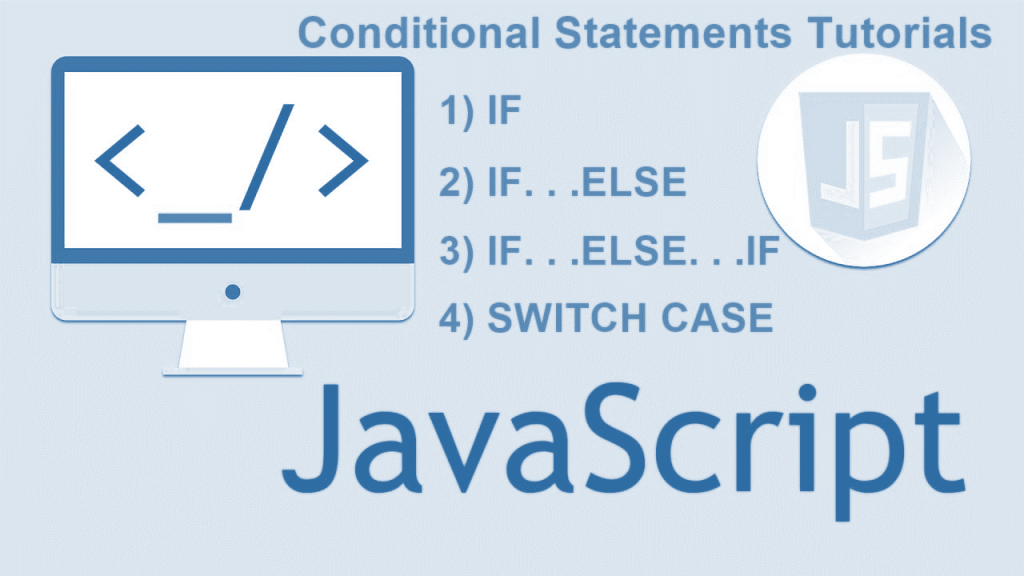
- If statement
- Else statement
- Else if statement
- Switch statement (You will learn about switch staement in next chapter)
The if Statement
If statement is used to specify a block of JavaScript code to be executed if the condition comes true. Its syntax is given below:
Remember that if the statement is in lower case, writing the uppercase letters (If or IF) will generate an error.
<!DOCTYPE html> <html> <body> <script> var m = 85; if (m > 70) { document.write("You are passed!"); } </script> </body> </html>
Output:

The else Statement
Else statement is used to specify a block of code to be executed if the condition comes false. Its syntax is given below:
Consider the below example to understand this concept:
<!DOCTYPE html> <html> <body> <script> var m = 32; if (m > 33) { document.write("You are passed!"); } else { document.write("You are failed!"); } </script> </body> </html>
Output:

The else if Statement
The else if statement is used to specify a new condition if the first condition comes false. Its syntax is given below:
Consider the below example to understand this concept:
<!DOCTYPE html> <html> <body> <script> var m = 55; if (m > 80) { document.write("Excellent!"); } else if (m > 50) { document.write("Average!"); } else { document.write("Below Average!"); } </script> </body> </html>
Output:
