The JavaScript comparison and Logical operators are important topics in JavaScript. Comparison operators compare two values and give back a Boolean value: either true
or false
.
Comparison operators can be used in conditional statements or in loop statements to compare values and take action depending on the result.
JavaScript Comparison Operators
To determine the equality or difference between JavaScript variables or values the JavaScript comparison operators are used in logical statements.
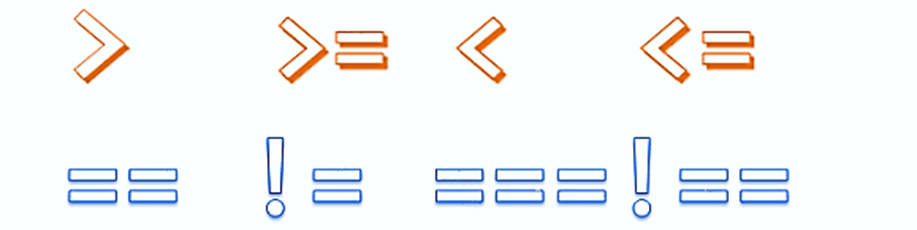
The table below explains the JavaScript comparison operators:
Operator | Name | Description |
== | Equal to | Return true if both side operands are equal |
!= | Not equal to | Return true if operands are not equal |
=== | Strict equal to | Return true if operands are equal and of the same type |
!== | Strict not equal to | Return true if operands are equal but of different types or not equal at all |
> | Greater than | Return true if left side operand is greater |
>= | Greater than or equal to | Return true if left side operand is greater or equal to the right |
< | Less than | Return true if right side operand is greater |
<= | Less than or equal to | Return true if right side operand is greater or equal to left |
Which statement can be used at which time depends on the comparison value and action to be taken.
JavaScript Logical Operators
To determine the logic between variables or values the logical operators are used. Given that x = 5
and y = 2
, the table below explains the logical operators:
Operator | Name | Description |
&& | and | It returns true if both side values are true |
|| | or | It returns true if either of the value is true |
! | not | It returns true if both side values are opposite |