PHP Data Types have been used to store various types of data or values. PHP supports eight primitive data types, which are further divided into three categories:
- Scalar Types (Predefined)
- Compound Types (user-defined)
- Special Types
PHP Data Types: Scalar Types
The Scalar types are also known as predefined data types. A scalar data type is the most fundamental data type, containing only one atomic value at a time. In PHP data types, there are 4 scalar data types.
- Integer
- Boolean
- Float
- String
Integer
Integers can only store whole numbers with either positive or negative signs, such as, numbers without a fractional part or a decimal point.
<?php $dec = 34; $oct = 0243; $hexa= 0x45; var_dump($dec); echo "</br>"; echo "Octal number: " .$oct. "</br>"; echo "HexaDecimal number: " .$hexa. "</br>"; ?>

In the above example the var_dump() is a predefined function, It returns the data type and value of variable.
Boolean
Booleans are the most basic data form, and they work similarly to switches. It can only have one of two values: TRUE (1) or FALSE (0).
It’s often used in conjunction with conditional statements. If the condition is true, it returns TRUE; if it is false, it returns FALSE.
Syntax
<?php
if (TRUE)
// You can print any message.
echo "The condition is TRUE.";
if (FALSE)
echo "The condition is FALSE.";
?>
Float
Float can hold numbers with a fractional or decimal point, as well as it can be negative or positive.
<!DOCTYPE html> <html> <body> <?php $val1 = 20.11; $val2 = 54.26; $sum = $val1 + $val2; var_dump($sum); ?> </body> </html>

String
String can store letters, numbers, and even special characters. It is basically non-numeric data type. During declaration, Strings are enclosed in double quotes. Single quotes can also be used to write strings, but they will be interpreted differently when printing variables.
Lets see an example to get it more clearly:
<?php $name = "Tutorials Art"; //Output of both single and double quote statements is different echo " Welcome to $name"; echo "</br>"; echo 'Welcome to $name'; ?>

PHP Data Types: Compound Types
The Compound types are also known as user-defined data types. The term “compound data type” refers to a data type that can contain many independent values. PHP data types has two compound types:
- Array
- Object
Array
An array can contain multiple values in a single variable .Lets see an example:
<h2> Example of Array</h2> <?php $lang= array("C","C++","Java"); echo "The var_dump() is a predefined function, It returns the data type and value of variable. </br> </br>"; var_dump($lang); echo "</br> </br>"; echo "Array Element1: $lang[0] </br>"; echo "Array Element2: $lang[1] </br>"; echo "Array Element3: $lang[2] </br>"; ?>

Object
Objects are instances of user-defined classes that can hold both values and functions. Individual objects inherit all of the properties and behavior of the class when they are created, but the properties will have different values for each object.
Objects are explicitly declared and created from the new keyword.
<h2> Example of object</h2> <?php class lang { function complang() { $lang_name = "PHP"; echo "language: " .$lang_name; } } // Object of class lang $obj = new lang(); // Now through this object function of class can be accessed. $obj -> complang(); ?>
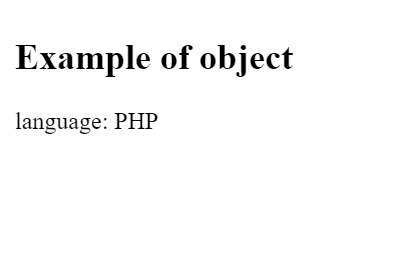
PHP Data Types: Special Types
PHP has two special types:
- Null
- Resource
Null Value
These are special variables that can only have one value, which is NULL. It is written in capital letters and it is case sensitive.
Note: When a variable is created, by default it is given the value NULL.
<h2> Example of Null Value</h2> <?php $nl = NULL; echo $nl; //it will not give any output echo "</br>"; echo 'As its Null So,Output is not shown above. Now lets check the var $nl type through function var- dump() </br> </br>'; var_dump($nl); ?>
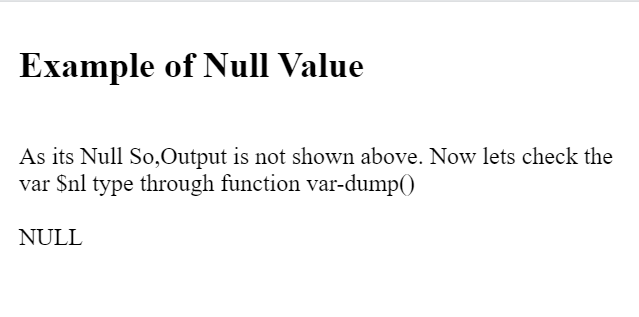
Resource
In PHP, resources are not the same as data types. These are mostly used to save the function calls and references to external PHP resources.
For Example: database call
Since this is a more advanced PHP topic, we’ll go over it in more detail with examples later on.