The PHP File Create/Write methods will be covered in this chapter.
PHP Create File – fopen()
A file can also be created using the fopen() method. Perhaps puzzlingly, in PHP, a file is created using the same function that opens files.
If a file with the same name already exists, fopen() will open it; otherwise, fopen() will create and open a new file for writing.
Syntax:
$myfile = fopen("filename.txt", "w")
The first parameter is filename and next is file mode which we have discussed in File open chapter.
Note: When creating a new file, the ‘a’ and ‘a+’ modes are cannot be used.
PHP Write to File – fwrite()
The fwrite() function is a built-in PHP function that allows you to write to an open file. The fwrite() function terminates when it reaches the end of the String you want to write or the length supplied as a parameter, whichever comes first.
Syntax:
fwrite(file, string, length)
Parameters of fwrite():
In PHP, the fwrite() method takes three parameters:
Parameter | Description |
file | This is a required argument that specifies the file |
string | It’s a required argument that provides the string that will be written. |
length | It is an optional parameter that sets the maximum number of bytes that can be written. |
Example:
<?php
$file = fopen("names.txt", "w") or die("Unable to open file!");
$txt = "John\n";
fwrite($file, $txt);
$txt = "Henry\n";
fwrite($file, $txt);
$txt = "Bean\n";
fwrite($file, $txt);
fclose($file);
?>
The created file will be look like this:
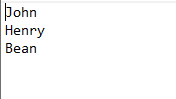
PHP Overwriting
We can now demonstrate what occurs when we open an existing file for writing now that “names.txt” contains some data. All old data will be ERASED, and a file will be formed.
<?php
$file = fopen("names.txt", "w") or die("Unable to open file!");
$txt = "Hana\n";
fwrite($file, $txt);
$txt = "Anna\n";
fwrite($file, $txt);
$txt = "Ben\n";
fwrite($file, $txt);
fclose($file);
?>
When we access the “names.txt” file now, all previous data has erased, leaving only the information we just entered:
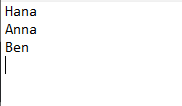