String can store letters, numbers, and even special characters. It is basically non-numeric data type. As we have discussed the String data type in PHP String.
In this chapter, we’ll look at some of the more commonly used PHP String functions.
PHP String Functions
Now lets discuss some built-in PHP string functions.
Built-in functions PHP string functions are some existing library functions that can be used directly in our programs making an appropriate call to them.
Functions | Description |
strlen() | It returns length of string. |
strrev() | It reverse a string. |
str_replace() | It replace the characters of string with other characters. |
strpos() | It search for the position of mentioned text. |
str_word_count() | It counts the number of words in String. |
str_repeat() | It is used to repeat string. |
strtoupper() | It converts string to upper case string. |
strtolower() | It converts string to lower case string. |
Now lets see example of these above PHP string functions:
String length Function
To measure the length of string , strlen() is used, it returns the length of string.
<h4>String Length Function Example</h4> <?php echo strlen("Tutorials art!"); ?>
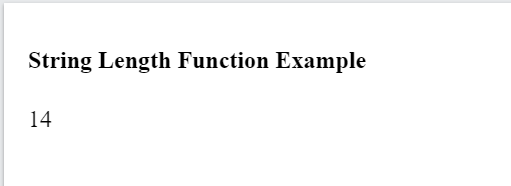
Reverse String Function
strrev() is used to reverse a string. This function takes a string as an argument and return its reversed string.
<h1>Reverse String Function Example</h1> <?php echo strrev(" Welcome to Tutorials art!"); ?>
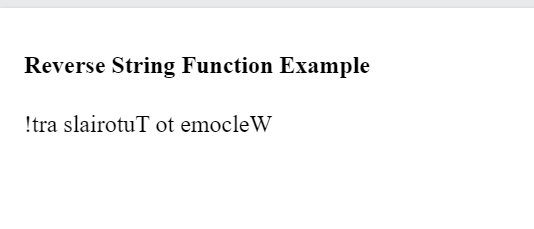
Replace String Function
The str_replace() function in PHP replaces certain characters in a string with other characters.
Three strings are passed as arguments to this function. The original string is returned as the third argument, and the first argument is replaced by the second. In other words, it substitutes the second argument for all occurrences of the first argument in the original string.
<h4>Replace String Function Example</h4> <?php //Original String echo"Welcome To tutorials art </br> </br>"; //After replace fuction value of String echo str_replace("To tutorials art", "back", "Welcome back!"); ?>

Search String Position
strpos() is used to search for the position of specific text. This function takes two string arguments, the first argument is the original string and the second argument is the string/text you want to search.
if the searched string is present in original string, than this function returns the position of searched string else, it returns false.
<h4>String Position Function Example</h4> <?php echo " The below function will search for letter a </br>"; echo strpos("Tutorials art!", "a"); echo "</br>"; echo "The below function will search for starting position of word art </br>"; echo strpos("Tutorials art!", "art"); ?>
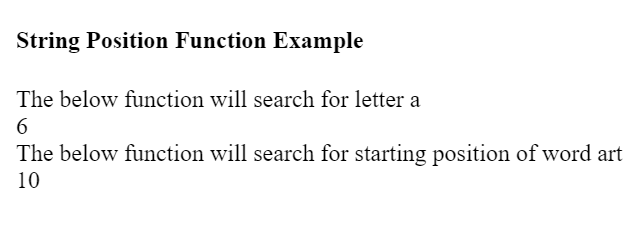
Note: The position of first character in a string starts with 0.
Word Count in String
str_word_count() is used to count the number of words in a String.
<h4>Word Count Example</h4> <?php echo str_word_count(" Welcome to Tutorials art!"); ?>
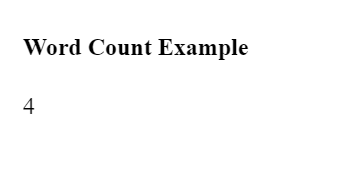
Repeat String Function
str_repeat() is used to repeat string.
<h4>Repeat Function Example</h4> <?php echo str_repeat("ha",4); ?>
String Uppercase Function
strtoupper() is used to convert a string into a uppercase string.
<h4>Uppercase Function Example</h4> <?php $str = "Welcome to Tutorials art"; echo strtoupper($str); ?>
String Lowercase Function
strtolower() is used to convert a string into lowercase string.
<h4>Lowercase Function Example</h4> <?php $str = "WELCOME TO TUTORIALS ART"; echo strtolower($str); ?>