The validation of form fields is demonstrated in this chapter. Let’s have a look at the form fields we’ll be creating.
Field | Validation Rules |
Name | Must only contain letters and whitespace |
Must contain a valid email address (with @ and .) | |
Website | It must contain a valid URL |
We discussed required fields and errors messages in the last chapter. Now we’ll look at the validation code for each field individually, and then we’ll look at the complete example.
Validate Name
The code below demonstrates a basic method for determining whether the name field only contains letters, dashes, apostrophes, and whitespaces. If the name field’s value is invalid, an error message is put away:
$name = check_input($_POST["name"]);
if (!preg_match("/^[a-zA-Z-' ]*$/",$name)) {
$nameError = "Invalid Pattern!!!! Only letters and white space allowed";
}
Note: The preg_match() function is used to match the input with defined patterns.
Validate Email
The filter_var() method in PHP is the simplest and safest approach to check whether an email address is valid. If the e-mail address is not valid, an error message is put away.
$email = check_input($_POST["email"]);
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$emailError = "Invalid email format!!! @ and .com missing ";
}
Validate URL
The code below demonstrates a basic method for determining whether the Website field contains a valid URL. If the value is invalid, an error message is put away:
$website = check_input($_POST["website"]);
if (!preg_match("/\b(?:(?:https?|ftp):\/\/|www\.)[-a-z0-9+&@#\/%?=~_|!:,.;]*[-a-z0-9+&@#\/%=~_|]/i",$website)) {
$websiteError = "Invalid URL";
}
PHP Forms – Field Validation Example
<!DOCTYPE HTML> <html> <head> <style> .error {color: #FF0000;} </style> </head> <body> <?php // define variables and set to empty values $nameError = $emailError = $genderError = $websiteError = ""; $name = $email = $gender = $comment = $website = ""; if ($_SERVER["REQUEST_METHOD"] == "POST") { if (empty($_POST["name"])) { $nameError = "Name is required"; } else { $name = test_input($_POST["name"]); // check if name only contains letters and whitespace if (!preg_match("/^[a-zA-Z-' ]*$/",$name)) { $nameError = "Only letters and white space allowed"; } } if (empty($_POST["email"])) { $emailError = "Email is required"; } else { $email = test_input($_POST["email"]); // check if e-mail address is well-formed if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $emailError = "Invalid email format"; } } if (empty($_POST["website"])) { $website = ""; } else { $website = test_input($_POST["website"]); // check if URL address syntax is valid if (!preg_match("/\b(?:(?:https?|ftp):\/\/|www\.)[-a-z0-9+&@#\/%?=~_|!:,.;]*[-a-z0-9+&@#\/%=~_|]/i",$website)) { $websiteError = "Invalid URL"; } } if (empty($_POST["comment"])) { $comment = ""; } else { $comment = check_input($_POST["comment"]); } if (empty($_POST["gender"])) { $genderError = "Gender is required"; } else { $gender = check_input($_POST["gender"]); } } function check_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } ?> <h2>PHP Form Validation Example</h2> <p><span class="error">* required field</span></p> <form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>"> Name: <input type="text" name="name"> <span class="error">* <?php echo $nameError;?></span> <br><br> E-mail: <input type="text" name="email"> <span class="error">* <?php echo $emailError;?></span> <br><br> Website: <input type="text" name="website"> <span class="error"><?php echo $websiteError;?></span> <br><br> Comment: <textarea name="comment" rows="5" cols="20"></textarea> <br><br> Gender: <input type="radio" name="gender" value="female">Female <input type="radio" name="gender" value="male">Male <input type="radio" name="gender" value="other">Other <span class="error">* <?php echo $genderError;?></span> <br><br> <input type="submit" name="submit" value="Submit"> </form> <?php echo "<h2>Your Input:</h2>"; echo $name; echo "<br>"; echo $email; echo "<br>"; echo $website; echo "<br>"; echo $comment; echo "<br>"; echo $gender; ?> </body> </html>
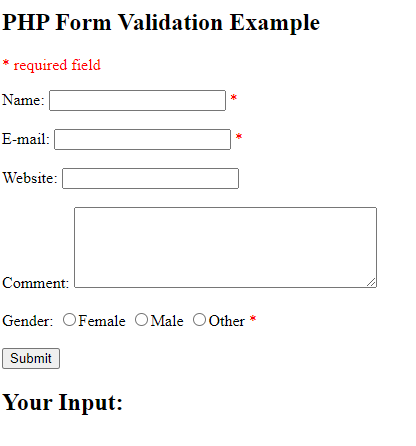
The next step is to demonstrate how to prevent the form from submitting with all input fields empty.