C# For Loop has similar functionality as while loop but with different syntax. For loops are preferred when the number of times loop statements are to be executed is known beforehand. The loop variable initialization, condition to be tested, and increment/decrement of the loop variable are done in one line in for loop thereby providing a shorter, easy to debug structure of looping.
A C# for loop is a repetition control structure that allows you to efficiently write a loop that needs to execute a specific number of times.
Syntax: C# For Loop
for ( init; condition; increment/decrement ) { statement(s); }
Graphical representation: C# For Loop
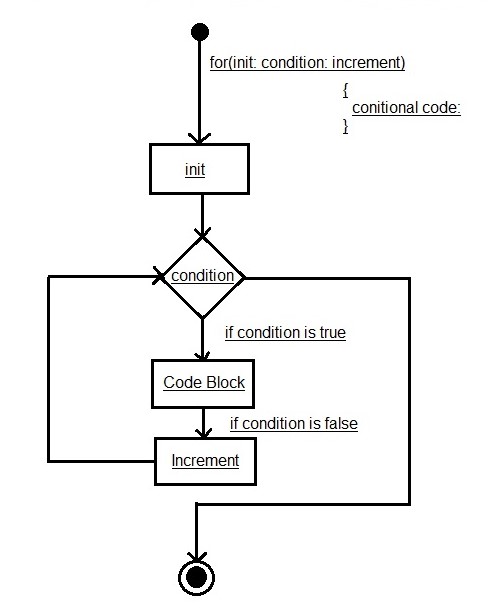
The for keyword, which signifies a for loop, is referred to as for.
init: Refers to the initializer expression, which is a variable that is used to control the loop (s). A loop control variable is a local variable whose value determines how the for loop condition is executed: This is a Boolean expression that refers to the condition expression. The loop control variable is used in this expression to determine whether the set of statements inside the for loop is performed or not.
The increment /decrement expression, which adds or decrements the value of the loop control variable, is referred to as update. The for loop executes this expression at the conclusion of each iteration. Iteration is the process of repeating a procedure in order to produce a (potentially unlimited) sequence of results. Each iteration of the process is defined as a single repetition of the process, with the output of each iteration serving as the starting point for the next iteration.
statements: Refers to the set of statements inside the for loop that is executed when the condition expression evaluates to true.
The initializer expression in C# For Loop is run first when the for loop is run. The condition expression is then executed after that. If an update expression is executed, The control returns to the condition expression, which is once again assessed. As long as the condition remains true, the process will continue. The for loop is finished when the condition becomes false, and control is passed to the statement that follows it. The initializer expression is the only one that runs once, although the condition and update expressions can run several times.
using System; namespace Loops { public class Program { public static void Main(string[] args) { /* for loop execution */ for (int x = 6; x < 12; x = x + 1) { Console.WriteLine("Value of X: {0}", x); } Console.ReadLine(); } } }
It’s worth noting that in C# For Loop, any or all of the init, condition, or increment /decrement expressions can be omitted. The semicolon that separates the three expressions, however, cannot be omitted. Only when you define and initialise the loop control variable before the for loop may you skip the init expression. If you leave out the, the programme will run indefinitely. Infinite loops are loops that keep running indefinitely. You may also make an unlimited loop by eliminating the for loop’s update expression. When writing programmes, however, you should avoid generating infinite loops.
C# Infinite for loop:
An infinite loop is the one which iterates infinite number of times. the for loop becomes an infinite loop if the condition provided is never going to be fulfilled.
C# infinite for loop Example:
If we put two semicolons only in for loop then the loop will iterate till infinite times. this is the simplest way to create an infinite for loop. The example is given below.
2 3 4 5 6 7 8 9 10 11 12 13 using System; public class InfiniteForLoopExample { public static void Main (string[] args) { for(;;) { Console.writeLine("this is an infinite loop"); } } }