Array in C# is used to store a fixed-size sequential collection of elements of the same data type, that is referred to by a common name. The length of the array specifies the number of elements in that array. And each data item in the array is known as the element of the array. Array in C# works differently than C or C++. Elements of the array are ordered and each element has an index starting from 0.
The following figure illustrates an array representation.
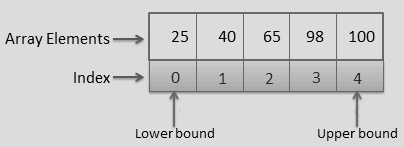
There are some important Points to Remember About C# Arrays:
- Array in C# is an object of base type System.Array.
- Arrays in C# are dynamically allocated.
- The elements of Array can be of any type.
- The default values of the numeric array are set to be zero.
- The types of arrays are reference types that are derived from the abstract base type Array.
- Arrays in C# are objects, their length can be found using member length.
- All the variables in the array are ordered. Each has an index beginning from 0.
Syntax :
< datatype > [ ] < name_array >
Declaring Array in C#:
To declare array in C# define the element type using <datatype>, <name_array> shows the name of array. [] specifies the size of the array.
Initializing Array in C#:
To assign Array in C#, the new keyword is created. By this keyword, an array can be declared and initialized at the same time. A new keyword is used because an array is a reference type.
Syntax of Initilizing Array:
datatype [ ] < Name_Array > = new < datatype > [size]; For Example: int[] Value = new int[5];
Assigning elements of Array in C#:
In Array, values can be assigned at the time of initialization. The other way to assign value is by using an index after the declaration and initialization. Through index, array values can be accessed.
See this example given below for declaration, initialization, and assigning value in Array.
#declaration & initializes: int[] intArray = new int[3]; #assigning value 5 on index 1 intArray[1] = 5;
Accessing elements of Array:
Indexing the array name is the way to access elements in an array. To access a specific value we have to place the index of the element within square brackets right after the name of the array.
For Example:
float value = value[9];
using System; namespace ArrayApplication { public class MyArray { public static void Main(string[] args) { int [] z = new int[7]; int m,n; /* initialize elements of array n */ for ( m = 0; m < 7; m++ ) { z[ m ] = m + 10; } /* output each array element's value */ for (n = 0; n < 7; n++ ) { Console.WriteLine("[{0}] Element = {1}", n, z[n]); } } } }
Types of Array in C#:
Following are the basic types of Arrays in C#.
1- Param Arrays:
This type of array is used to pass an unknown number of parameters to a function.
using System; namespace ArrayApplication { public class ParamArray { public int AddElements(params int[] arr) { int Add = 0; foreach (int x in arr) { Add += x; } return Add; } } public class TestClass { public static void Main(string[] args) { ParamArray app = new ParamArray(); int Add = app.AddElements(68, 129, 198,984,1920); Console.WriteLine("The Total Sum is: {0}", Add); } } }
2- Jagged Arrays:
C# supports multidimensional arrays, which are arrays of arrays.
using System; namespace ArrayApplication { public class MyArray { public static void Main(string[] args) { /* showing array of 5 array of integers*/ int[][] x= new int[][]{new int[]{0,0},new int[]{2,1}, new int[]{5,4},new int[]{ 7, 5} , new int[]{9,6}}; int m, n; /* output each array element's value */ for (m= 0; m< 5; m++) { for (n= 0; n < 2; n++) { Console.WriteLine("x[{0}][{1}] = {2}", m, n, x[m][n]); } } } } }
3- Passing Arrays to Functions
You can pass to the function a pointer to an array by specifying the array’s name without an index.
using System; namespace ArrayApplication { public class MyArray { double getAverage(int[] arr, int size) { int x; double avg; int sum = 0; for (x = 0; x < size; ++x) { sum += arr[x]; } avg = (double)sum / size; return avg; } public static void Main(string[] args) { MyArray app = new MyArray(); /* int array with elements */ int [] balance = new int[]{5, 23, 178, 2050}; double avg; /* pass pointer to the array as an argument */ avg = app.getAverage(balance, 4 ) ; /* output the returned value */ Console.WriteLine( "Average value is: {0} ", avg ); } } }
4- Multi-dimensional Arrays:
C# supports multidimensional arrays which are two-dimensional arrays. The multi-dimensional array declaration, initialization, and accessing is shown below:
#Creating two- dimentional array of 2 columns and 3 rows int[, ] intarray = new int[3, 2]; #Creating a three-dimensional array of, 2, 3, and 4 int[,, ] intarray1 = new int[2, 3, 4];
using System; namespace ArrayApplication { public class MyArray { public static void Main(string[] args) { int[,] x = new int[4, 2] {{0,0}, {1,2}, {2,4}, {3,6} }; int i, j; /* output each array element's value */ for (i = 0; i < 4; i++) { for (j = 0; j < 2; j++) { Console.WriteLine("x[{0},{1}] = {2}", i, j, x[i,j]); } } } } }