C# Operators allow performing operations on variables and values (operands). Basically, Operators are the foundation of any programming language. Without them any programming language is incomplete. C# provides a number of operators. Operators knew as a symbol that defines what kind of operation needs to perform on operands.
Following are the type of built-in C# Operators:
- Arithmetic Operators
- Logical Operators
- Relational Operators
- Assignment Operators
- Conditional Operators
- Bitwise Operators
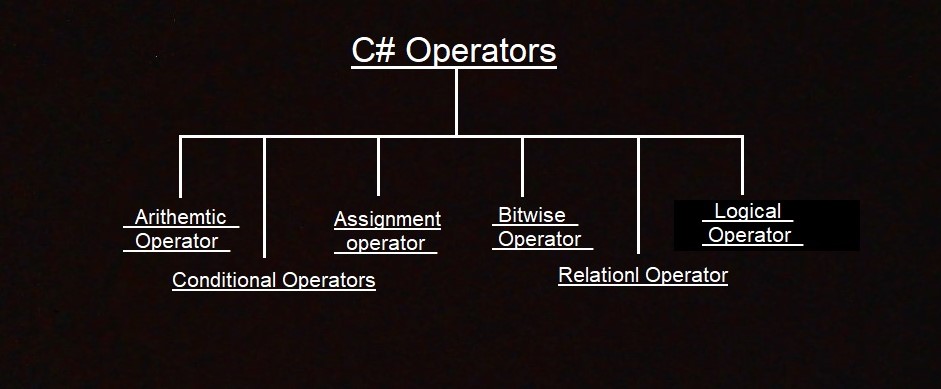
Arithmetic Operators: C# Operators
Arithmetic C# Operators, are basically useful to perform basic arithmetic calculations or operations like addition, subtraction, division, etc on operators or variables.
Syntax:
Following are the main types of Arithmetic operators:
Operators | Description | Syntax |
+ Addition | Adds two operands. | x + y |
– Subtraction | Subtracts two operands. | x – y |
* Multiplication | Multiplies two operands. | x * y |
/ Division | Divides the first operand by the second. | x / y |
// Floor Division | Divides the first operand by the second. | x // y |
% Modulus | Returns the remainder when the first operand is divided by the second. | x % y |
** Power/Exponent | Returns first raised to power second. | x ** y |
using System; namespace OperatorsAppl { public class Program { public static void Main(string[] args) { int x = 14; int y = 29; int z; z = x + y; Console.WriteLine("Value of Z is {0}", z); z = x - y; Console.WriteLine("Value of Z is {0}", z); z = x * y; Console.WriteLine("Value of Z is {0}", z); z = x / y; Console.WriteLine("Value of Z is {0}", z); z = x % y; Console.WriteLine("Value of Z is {0}", z); z = x++; Console.WriteLine("Value of Z is {0}", z); z = x--; Console.WriteLine("Value of Z is {0}", z); Console.ReadLine(); } } }
Logical Operators:
Logical operators are used to combine two or more conditions in C# Operators. It can be used to complement the evaluation of the original condition.
There are 3 logical C# Operators:
- Logical AND
- Logical OR
- Logical NOT
Operators | Description | Syntax |
Logical And ( && ) | Logical And= True if both the operands are true. | A && B |
Logical Or ( || ) | Logical Or= True if either of the operands is true | A II B |
Logical Not ( ! ) | Logical Not= True if the operand is false | !A |
using System; namespace OperatorsAppl { public class Program { public static void Main(string[] args) { bool x = true; bool y = true; if (x && y) { Console.WriteLine("Condition is true"); } if (x || y) { Console.WriteLine("Condition is true"); } /* lets change the value of x and y */ x = false; y = true; if (x && y) { Console.WriteLine("Condition is true"); } else { Console.WriteLine("Condition is not true"); } if (!(x && y)) { Console.WriteLine("Condition is true"); } Console.ReadLine(); } } }
Relational Operators:
Relational C# Operatorsare used to comparing any two values, to check the relation between two operands in C# Operators.
Following are the main types of Relational operators:
Operator | Description | Syntax |
---|---|---|
> | It checks if the value of the left operand is greater than the value of the right operand. | (A > B) |
< | It checks if the value of the left operand is less than the value of the right operand. | (A < B) |
!= | It checks if the values of two operands are equal or not, if not equal then the condition becomes true. | (A != B) |
== | It checks if the values of two operands are equal or not, if yes then the condition becomes true. | (A == B) |
<= | It checks if the value of the left operand is less or equal to the value of the right operand. | (A <= B) |
>= | It checks if the value of the left operand is greater or equal to the value of the right operand. | (A >= B) |
using System; public class Program { public static void Main(string[] args) { int x = 9; int y = 7; if (x == y) { Console.WriteLine("x is equal to y"); } else { Console.WriteLine("x is not equal to y"); } if (x < y) { Console.WriteLine("x is less than y"); } else { Console.WriteLine("x is not less than y"); } if (x > y) { Console.WriteLine("x is greater than y"); } else { Console.WriteLine("x is not greater than y"); } /* Lets change value of x and y */ x = 14; y = 22; if (x <= y) { Console.WriteLine("x is either less than or equal to y"); } if (y >= x) { Console.WriteLine("y is either greater than or equal to x"); } } }
Assignment Operators:
Assignment operators in C# Operators, are utilized to assigning a value to a variable, in which the left side of the assignment operator shows the variable and the right side shows the value. The variable and value should have the same data type, otherwise, the compiler will raise an error.
Given below are the different types of assignment operators:
Operator | Description | Example |
= | Shows the Simple assignment operator | Z= x + y assigns value of x + y to Z |
+= | Shows the Add AND assignment operator | x+= y defines as x = x + y |
-= | Shows the Subtract AND assignment operator | x-= y defines as x = x – y |
*= | Shows the Multiply AND assignment operator | x *= y defines as x = x * y |
/= | Shows the Divide AND assignment operator | x /= y means x = x / y |
%= | Shows the Modulus AND assignment operator | x%= y means x = x % y |
&= | Shows the Bitwise AND assignment operator | x&= 2 is same as x = x&2 |
^= | Shows the Bitwise exclusive OR and assignment operator | x^= 2 is same as x = x ^ 2 |
>>= | Shows the Right shift AND assignment operator | x >>= 2 is same as x = x >> 2 |
<<= | Shows the Left shift AND assignment operator | x<<= 2 is same as x = x << 2 |
|= | Bitwise inclusive OR and assignment operator | x |= 2 is same as x = x | 2 |
using System; namespace OperatorsAppl { public class Program { public static void Main(string[] args) { int x = 76; int y; int z; z = x; Console.WriteLine("Value of z = {0}", z); z += x; Console.WriteLine("+= Value of z = {0}", z); z -= x; Console.WriteLine("-= Value of z = {0}", z); z *= x; Console.WriteLine("*= Value of z = {0}", z); z /= x; Console.WriteLine("/= Value of c = {0}", z); z = 200; z %= x; Console.WriteLine("%= Value of c = {0}", z); z <<= 2; Console.WriteLine("<<= Value of c = {0}", z); z >>= 2; Console.WriteLine(">>= Value of c = {0}", z); z &= 2; Console.WriteLine("&= Value of c = {0}", z); z ^= 2; Console.WriteLine("^= Value of c = {0}", z); z |= 2; Console.WriteLine("|= Value of c = {0}", z); Console.ReadLine(); } } }
Conditional Operators:
Conditional operator in C# Operators, considered as a shorthand version of if-else statement. It contains 3 operands. It will return one of two values that depends on the value of a Boolean expression.
Syntax is given below:
Syntax:
Values used in the above Syntax are explaned below:
- Condition is used to evaluate between given expressions either true or false.
If the given condition is true,
The first_expression is evaluated and shows as the result. And if the given condition is false,
The second_expression is evaluated and shows as the result.
// C# program of Conditional Operator using System; namespace Conditional { public class Csharp { // Main Function public static void Main(string[] args) { int x = 7, y = 31, result; // Using Conditional Operator To find which value is greater result = x > y ? x : y; // To display the result Console.WriteLine("Result: " + result); // Using Conditional Operator to find which value is greater result = x < y ? x : y; // To display the result Console.WriteLine("Result: " + result); } } }
Bitwise Operators:
Bitwise operators works on bits and perform bit by bit operation. Description is given below,
Bitwise Operators | Description | Syntax | Example (a = 0, b = 1) |
& (bitwise AND) | It takes two numbers as operands and perform AND on every bit of two numbers. AND = 1, only if both bits are 1. | a & b | a & b (0) |
^ (bitwise XOR) | It takes two numbers as operands and performs XOR on every bit of two numbers. XOR = 1, if the two bits are different. | a ^ b | a ^ b (1) |
| (bitwise OR) | It takes two numbers as operands and perform OR on every bit of two numbers. OR=1, if any of the two bits is 1. | a | b | a | b (1) |
>> (right shift) | It takes two numbers, right shifts the bits of the first operand, the second operand decides the number of places to shift. | b >> 2 | b >> 2 (001) |
<< (left shift) | It takes two numbers, left shifts the bits of the first the operand, the second operand decides the number of places to shift. | b << 2 | b << 2 (100) |
using System; namespace OperatorsAppl { public class Program { public static void Main(string[] args) { int x = 34; int y = 17; int z = 0; z = x & y; Console.WriteLine(" Value of z is {0}", z ); z = x | y; Console.WriteLine(" Value of z is {0}", z); z = x ^ y; Console.WriteLine(" Value of z is {0}", z); z = ~x; Console.WriteLine(" Value of z is {0}", z); z = x << 2; Console.WriteLine(" Value of z is {0}", z); z = x >> 2; Console.WriteLine(" Value of z is {0}", z); Console.ReadLine(); } } }