C# Attributes are very useful in a programming language. In C# attributes are used to bring metadata about various code elements which include methods, properties, and types. They can be placed on certain entities in the source code to specify additional information. To add attributes in the code ([ ]) are used at the top of the code element.
Attributes have been part of .NET since the beginning. As such, they were created before generics were introduced and never quite caught up, which means if you want to refer to a type in the attribute, you must expose a Type parameter or property.
Simply put, attributes are metadata tags that hold some information. The syntax for them is the type name in square brackets above the code being tagged, like so:
They can be attached to almost anything, including classes, methods, fields, structs, and types. They can even be provided parameters to offer accessible input, however they are limited to basic types. By executing the attribute as a method, you can set parameters:
Marking fields for serialisation is a typical use case. Many third-party libraries will implement their own tags, and C# provides a built-in [Serializable] tag that facilitates serialising a class to bytes. The C# driver for MongoDB, for example, includes a number of tags for serialising to Bson, as well as a specific tag that interprets a string as the document ID.
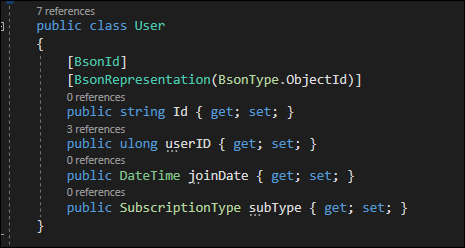
Properties of Attributes
- In C# attributes are derived from the System.Attribute Class.
- In C# attributes can have zero or more parameters.
- Just like methods, properties Attributes can also have arguments.
- All the code elements can have multiple attributes.
- To obtain the metadata of any program, the reflection process can be used.
Attributes Type:
There are 2 types of C# Attributes:
- Predefined Attributes
- Custom Attributes
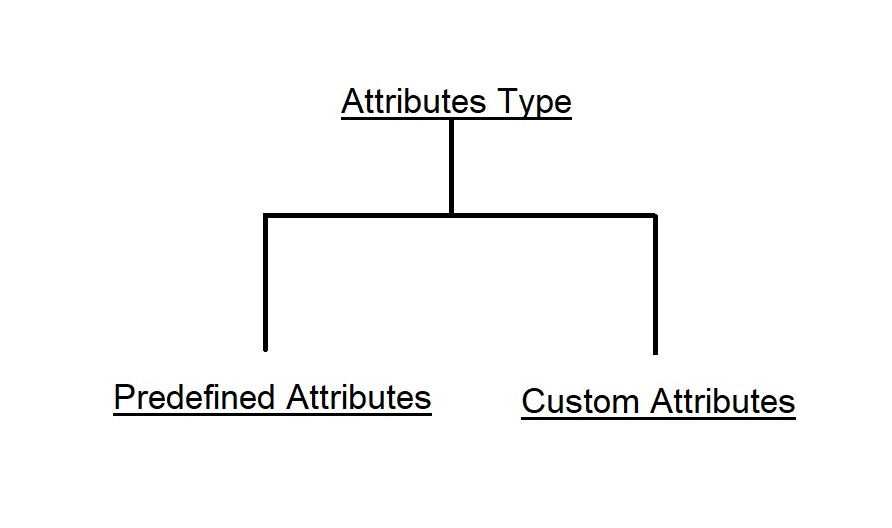
1- Predefined Attributes:
There are 3 types of predefined attributes:
Predefined Attributes | Description |
Obsolete | This predefined attribute spots the code elements that are not in use. |
Conditional | This predefined attribute defines the conditional method in which execution depends on a specified preprocessing identifier. |
AttributeUsage | This predefined attribute describes how a custom attribute class can be used. |
2- Custom Attributes:
Attributes can also be created in C#, those attributes are known as Custom attributes. These are created to attach declarative information to code elements. The extensibility of the .NET framework can be increased through custom attributes.
To create the custom attributes first we have to define the class that is derived from System.Attribute class. This class should have the suffix Attribute name. To define the usage of the creation of the custom attribute class, we use AttributeUsage attributes. Then create the constructor and the accessible properties of the custom attribute class.
How To Make Your Own Attributes
Of course, you can design your own custom attributes to apply to your classes with relative ease. It’s as simple as creating a class that extends System.Attribute. You can optionally provide this class a property that specifies how it should be used, such as only applying to classes or structs.
Then you may define fields and a function Object() { [native code] } that will populate the fields using the input parameters. This attribute works in the same way as new Attribute(params), but without the new keyword.
[System.AttributeUsage(System.AttributeTargets.Class | System.AttributeTargets.Struct)] public class AuthorAttribute : System.Attribute { private string name; public double version; public AuthorAttribute(string name) { this.name = name; version = 1.0; } }
Then you can use them to tag your code as long as the attribute is referenced in the marked code.
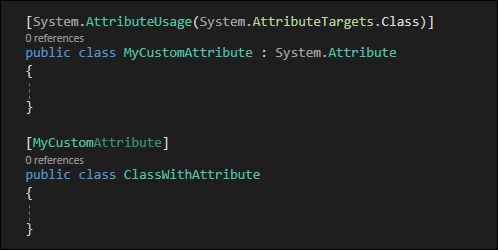
You will need to use reflection to access attributes programmatically. Reflection isn’t excellent for performance, but that usually doesn’t matter in the context of attribute use cases. Attribute is an option. Return an Attribute[, passing in the class type: GetCustomAttributes