In C# while loop, the loops keep repeating through a block of code until the specified condition is not True.
The loop has the test condition at the beginning of the loop. All the given statements are executed till the given boolean condition satisfies when the condition becomes false, the control will be out from the while loop.
Note that the loop body might not run at all, since the boolean condition is evaluated before the very first iteration of the while loop.
The syntax to declare a while loop is simply the while
keyword followed by a boolean condition in parentheses.
Syntax:
Graphical representation: C# While Loop
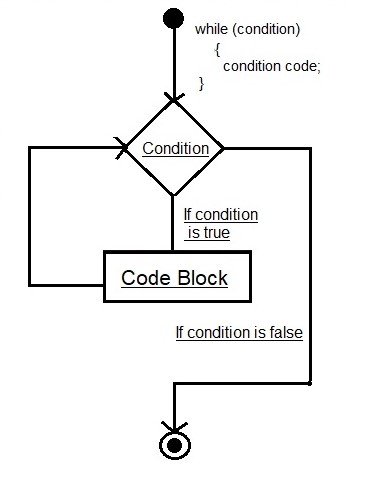
using System; namespace Loops { public class Program { public static void Main(string[] args) { /* local variable definition */ int x = 6; /* while loop execution */ while (x < 11) { Console.WriteLine("Values of x: {0}", x); x++; } Console.ReadLine(); } }