The C# Namespaces is a group of linked objects or types with the same name. If you don’t specify a namespace explicitly, the C# compiler creates one for you. The global namespace is the default namespace. You can’t modify the fact that all namespaces are implicitly public. Modifiers and attributes are not supported by a namespace declaration.
Namespaces in C# are used to permit you to create a system to manage your code in a group. Namespaces are also known as the way to keep one set of names separate from another set of names. The class names declared in one namespace can’t conflict with the same class names declared in another.
In large projects, namespaces are typically used to arrange the many classes and functions. We’ve been printing output on the console with the Console.WiteLine() statement since the beginning of this lesson. At the top of each programme, we also utilised the using System directive. System is a namespace, and Console is a class in the System namespace, and we stated this at the top with the use keyword to avoid writing the same namespace several times, such as System.Console.WriteLine().
Defining a C# Namespaces:
The syntax given below shows how to define namespaces in C#:
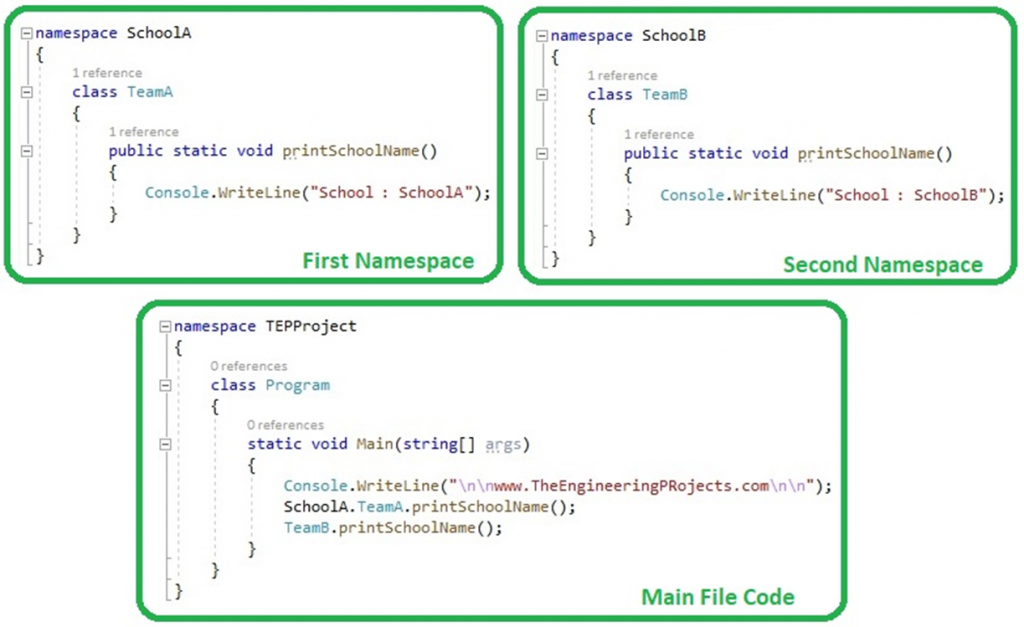
using System; namespace SchoolA{ public class namespace_csharp { public void func() { Console.WriteLine("School: School A"); } } } namespace SchoolB{ public class namespace_csharp { public void func() { Console.WriteLine("School: School B"); } } } public class TEPProject{ public static void Main(string[] args) { SchoolA.namespace_csharp fc = new SchoolA.namespace_csharp(); SchoolB.namespace_csharp sc = new SchoolB.namespace_csharp(); fc.func(); sc.func(); } }
Nested Namespaces:
Namespaces can also be defined inside of another namespace. It is called Nested Namespaces.
Defining Nested Namespaces:
The syntax for nested namespaces is given below:
namespace namespace_name1 { // code declarations namespace namespace_name2 { // code declarations } }
using System; using first_namespace; using first_namespace.second_namespace; namespace first_namespace { public class a { public void func() { Console.WriteLine("First Namespace"); } } namespace second_namespace { public class b { public void func() { Console.WriteLine("Second Namespace"); } } } } public class TestClass { public static void Main(string[] args) { a fc = new a(); b sc = new b(); fc.func(); sc.func(); } }