C# Exception handling is defined as the way to tell the program to move on to the next block of code or provide the defined result in certain situations. An exception is considered as the problem that arises during the execution of a program. It occurs when an exceptional situation happens in the program. In C# Exceptions provide a way to transfer control from one part of a program to another.
Keywords:
An Exception handling in C# is built upon 4 basic Keywords:
- try
- catch
- finally
- throw
Let’s discuss these keywords in detail:
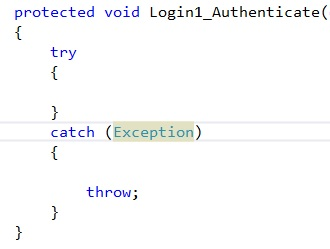
1- try:
A try block identifies a block of code for which particular exceptions are activated. try block is followed by one or more catch blocks. Try block will show compiler error if it’s used without finally and catch block.
The example given below will show the try-catch statement:
2- catch:
The catch keyword indicates the catching of an exception. A program catches an exception with an exception handler at the place in a program where you want to handle the problem.
The example given below will show the try-catch statement:
3- finally:
The finally block is used to execute a given set of statements, whether an exception is thrown or not thrown.
The example given below will show the try-finally statement:
4- throw:
This throw keyword is used because a program throws an exception when a problem shows up. An object can also be thrown if it is derived from the System.Exception class. A throw block statement is used to throw a present object in the catch block.
Catch(Exception e) { ... Throw e }
Syntax: C# Exception Handling
Exception Handling:
C# Exception Handling can be done by providing a structured solution. C# handles the exception in the form of try and catch blocks. C# uses the try, catch and finally block for error handling blocks. These blocks make the core program statements separated from the error-handling statements.
#Csharp exception handling using System; namespace ErrorHandlingApplication { public class DivideValues { int Answer; DivideValues() { Answer = 0; } public void division(int V1, int V2) { try { Answer = V1 / V2; } catch (DivideByZeroException e) { Console.WriteLine("Exception being caught: {0}", e); } finally { Console.WriteLine("Answer: {0}", Answer); } } public static void Main(string[] args) { DivideValues d = new DivideValues(); d.division(34, 0); } } }
Exception Classes in C#:
Exceptions are represented by the Classes. In C# The exception classes are derived from the System.Exception class.
Some exceptions classes are given below:
Exception Class | Description |
---|---|
System.IO.IOException | This exception class handles I/O errors. |
System.InvalidCastException | This exception class handles errors generated during typecasting. |
System.StackOverflowException | This exception class handles errors generated from stack overflow. |
System.OutOfMemoryException | This exception class handles errors generated from insufficient free memory. |
System.NullReferenceException | This exception class handles errors generated from referencing a null object. |
System.ArrayTypeMismatchException | This exception class handles errors are generated when the type is mismatched with the array type. |
System.DivideByZeroException | This exception class handles errors generated from dividing a dividend with zero. |
System.IndexOutOfRangeException | This exception class handles errors are generated when a method refers to an array index out of range. |