C# Switch Statement allows a variable to be tested for equality against a list of values. In the switch statement, each value is known as a case, and the variable which is being switched on is checked for each switch case.
The switch statement is also known as long if-else-if ladders. The given expression is checked for different cases, and only one match is executed. There is a statement used to move out of the switch, known as the Break statement. If we do not use the break statement, the control will flow to all cases below it until the break is found or the switch comes to an end. There is an optional default case at the end of the switch if none of the cases matches then the default case is executed.
Graphical representation of C# Switch Statement
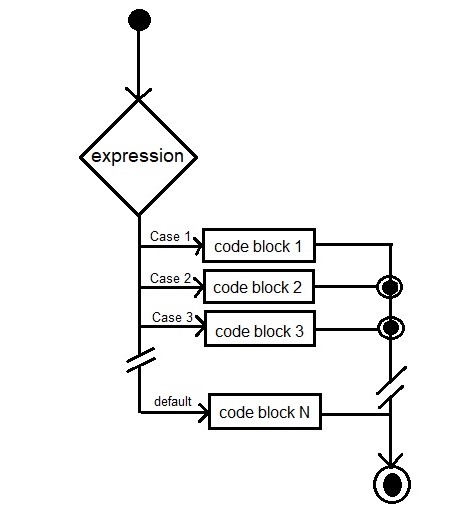
Control cannot pass from one switch section to the next within a switch statement. To pass control out of a switch statement, you normally use the break statement at the end of each switch section, as shown in the examples in this section. Return and throw statements can also be used to pass control out of a switch statement. The goto statement can be used to mimic the fall-through behaviour and pass control to another switch section.
The switch expression can be used in an expression context to evaluate a single expression from a list of candidate expressions based on a pattern match.
Syntax for C# Switch Statement
The following example will illustrate C# nested-switch statement.
using System; namespace DecisionMaking { public class Program { public static void Main(string[] args) { /* local variable definition */ int ID = '1'; switch (ID) { case 'A': Console.WriteLine("FIRST!"); break; case 'B': Console.WriteLine("SECOND"); break; case 'C': Console.WriteLine("THIRD"); break; default: Console.WriteLine("Invalid ID"); break; } Console.WriteLine("Your ID is {0}", ID); Console.ReadLine(); } } }