Unsafe code in C# can also be defined as Unverifiable code. These unsafe codes can be run outside the control of the Common Language Runtime (CLR) of the .NET frameworks. The “unsafe” keyword is used to tell the compiler that the control of this particular code will be done by the programmer. In an unsafe context, code uses pointers, allocate, and free blocks of memory. It calls methods using function pointers. Unsafe codes in C# are not necessarily dangerous. It’s just code whose safety cannot be verified. When we are using pointers, we should execute our program in unsafe mode.
Syntax:
The syntax for unsafe code declaration is given below:
unsafe context_declaration
Following subprograms can be made as Unsafe Code by the programmer:
- Class
- Types
- Struct
- Methods
- Code blocks
Compiling Unsafe Code
The example given below shows a program that is compiled with safe mode.
Example:
public static void Main(string[] args) { int xy = 56; int* z = &xy; Console.WriteLine("Result of XY is {0}", *z); Console.ReadLine(); }
The code will show following error:
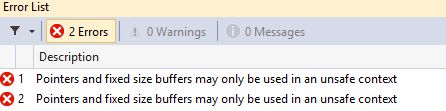
To compile unsafe code in C#, there is this /unsafe command-line switch that has to be specified with the command-line compiler. If we are using Visual Studio IDE then you need to enable the use of unsafe code in the project properties.
Follow the steps given below to enable unsafe mode in the project:
First, you have to open the option Project Properties by double-clicking the properties node in the solution explorer.
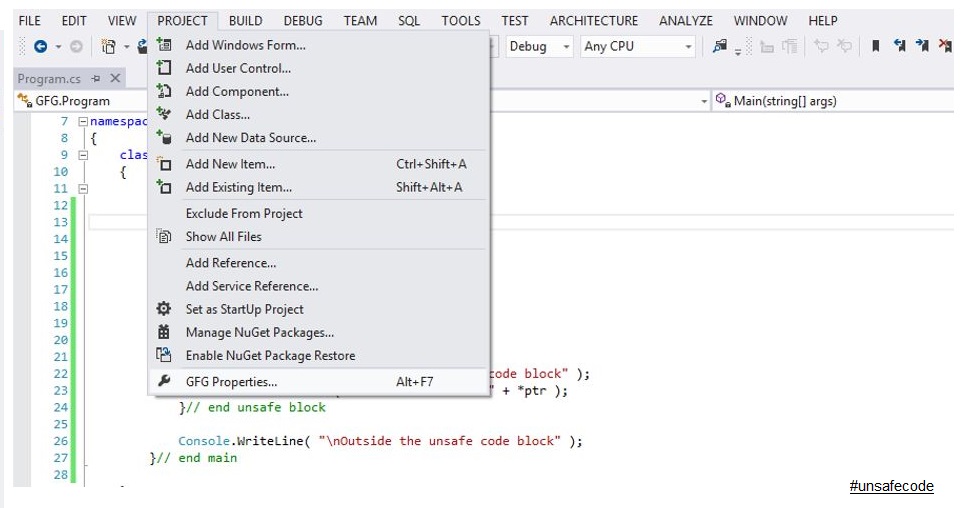
Then click on the Built tab given. Select the Allow Unsafe Code option to proceed.
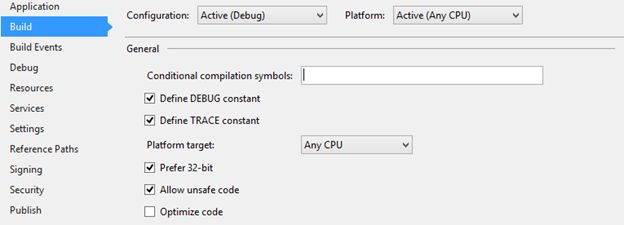
Pointers
A pointer is a variable whose value is the address of another variable i.e., the direct address of the memory location. similar to any variable or constant, you must declare a pointer before you can use it to store any variable address.
The general form of a pointer declaration is −
type *var-name;
Retrieving Data Values using Pointers:
A Pointer is a variable whose value is the address of another variable just like the direct address of the memory location. In C# stored data values can be retrieved at the location referenced by the pointer variables. To retrieve we have to use the ToString() method.
Example:
The example given below shows the retrieving data values:
using System; namespace UnsafeCode { public class Program { public static void Main() { unsafe { int value = 45; int* x = &value; Console.WriteLine("Data value is: {0} " , value); Console.WriteLine("Data value is: {0} " , x->ToString()); Console.WriteLine("Given address is: {0} " , (int)x); } } } }