C# Delegates are the reference type variables that hold the reference to a method. Delegates are similar to pointers to functions, in C or C++. The reference given to delegates can be changed at runtime. All delegates are implicitly derived from the System.Delegate class. They are used for implementing events and call-back methods.
Declaring Delegates in C#
While declaring Delegates, it determines the methods that can be referenced by the delegate. A delegate can refer to a method, having the same signature as the delegate.
public delegate int MyDelegate (string s);
Syntax for Delegates declaration:
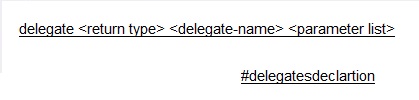
Using Delegates in C#:
The delegate printString can be used as to reference method that takes a string as input and returns nothing. Delegates can be used to call two methods. One can be used to print the string to the console, and the second one is used to print it to the file.
using System; using System.IO; namespace DelegateUse { public class PrintString { public static FileStream fs; public static StreamWriter sw; // delegate declaration public delegate void printString(string s); // this method prints to the console public static void WriteToScreen(string str) { Console.WriteLine("Delegates", str); } //this method prints to a file public static void WriteToFile(string s) { fs = new FileStream("c:\\message.txt", FileMode.Append, FileAccess.Write); sw = new StreamWriter(fs); sw.WriteLine(s); sw.Flush(); sw.Close(); fs.Close(); } // this method takes the delegate as parameter and uses it to // call the methods as required public static void sendString(printString ps) { ps("CSharp"); } public static void Main(string[] args) { printString ps1 = new printString(WriteToScreen); printString ps2 = new printString(WriteToFile); sendString(ps1); sendString(ps2); } } }
Mutlicasting Delegates
In C# to compose the Delegate objects, the “+” operator is used. The same type of delegates can be composed. A composed delegate object calls the two delegates from which it was composed. The “-” operator can be used to remove a component delegate from a composed delegate. Multicasting of Delegates can be defined as the property of delegates by which we can create an invocation list of methods that will be called when a delegate is invoked.
using System; delegate int NumberChanger(int x); namespace Delegate { public class TestDelegate { public static int value = 45; public static int AddNum(int y) { value += y; return value; } public static int MultNum(int z) { value *= z; return value; } public static int getNum() { return value; } public static void Main(string[] args) { //create delegate instances NumberChanger nc; NumberChanger nc1 = new NumberChanger(AddNum); NumberChanger nc2 = new NumberChanger(MultNum); nc = nc1; nc += nc2; //calling multicast nc(5); Console.WriteLine("Total Value = {0}", getNum()); } } }