The C# do-while loop is similar to the while loop. But there is one difference that the do-while loop checks the condition after executing the statements.
Syntax:
Graphical representation: C# do-while loop
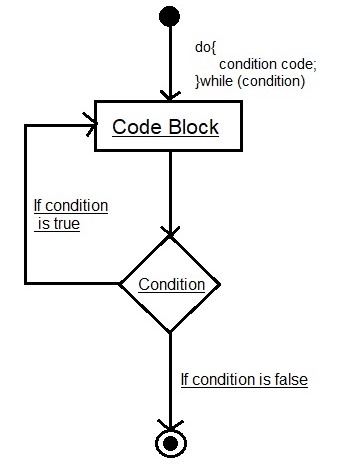
The control returns to the beginning of the loop if the condition is true. The control exits the loop when the condition is false. This means that before the condition is tested, the loop’s statements are executed. In all situations when the loop body must be executed at least once, the do while loop should be used. We often need a do while loop in a menu-driven software when activities are supposed to be repeated based on user input. When the user input is comparable to an exit command, the control will break out of the loop.
using System; namespace Loops { public class Program { public static void Main(string[] args) { /* local variable definition */ int x = 24; /* do loop execution */ do { Console.WriteLine("Value of x: {0}", x); x = x + 1; } while (x < 30); Console.ReadLine(); } } }
While and For Loops: What’s the Difference?
The two sorts of loops used to iterate over a set number of records or values are while and for loops. The trigger that closes the loop is the distinction between the two types. The for and foreach loops repeat over a set number of records or values until all of them have been accounted for. While and do while loops go over a set of values until a specified condition is met, therefore not every record is considered. This condition can be a changing flag, a changing value, or you can use the while and do while loops in the same way as the for loops to leave once a specific number of loops have been completed.
While and Do While Loops: What’s the Difference?
Understanding how code executes and manipulates values is required for software engineering. To correctly architect the code design, you must understand the complexities. As a result, it’s critical to know the distinction between the two forms of while loops.
The while loop runs until a condition is met first. This condition can be determined statically or dynamically in the code. While, on the other hand, always runs the code logic at least once. The “do” keyword is used to start the loop, after which the logic is executed and the condition is assessed. Because the condition is assessed first in a while loop, the code logic may never be executed.
To put it another way, the do while loop is always executed at least once, whereas the while loop logic may result in the loop never being executed. This may seem small, but it’s crucial when programming logic.
The do while loop, like other loops, can be exited early. When you want to escape the loop from a secondary condition, you normally do this.
Do while loops are useful when you need to run a piece of code at least once. For example, if you wish to add a set of numbers and there is always at least one value to add, you may use the do while loop to aggregate these numbers until all of the values are added. The sum is subsequently stored in a variable and displayed to the user.