The variable in the R language has a data type. All data types in R programming need some memory and have some operations that can be performed over it. We have the following data types in R programming:
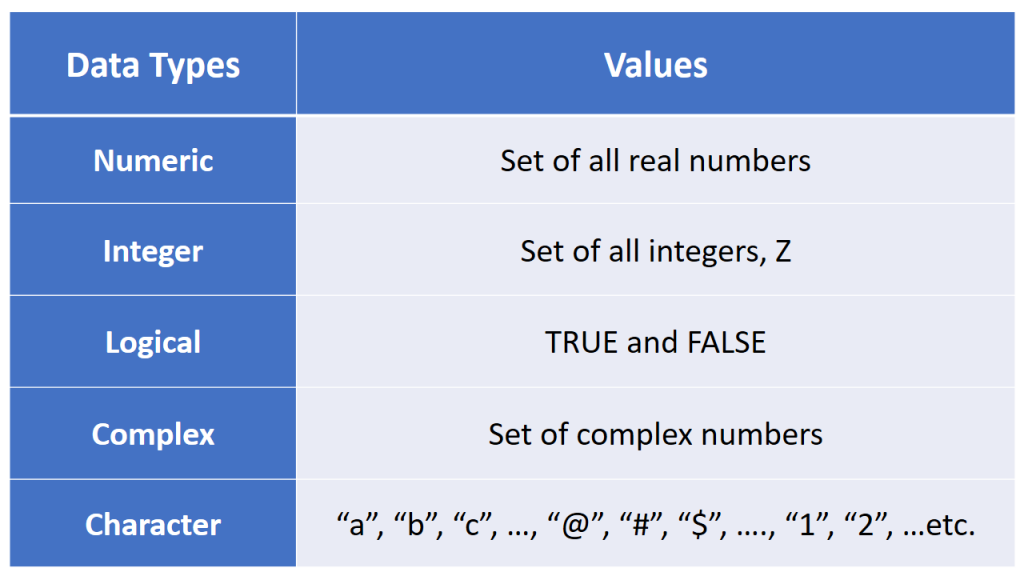
1. Numeric data type in R
The decimal values in the R language are called numeric. It is used as a default data types in R for numbers. If we assign a decimal value to a variable, it will be of numeric data type.
2. Integer data type in R
The set of all integers is called integer data types in the R language. We can create a new integer as well as covert a value into integer data type in R language.
3. Logical data type in R
The Boolean value is either true or false is known as a logical data type in R language. The logical data type is created using a comparison operator between variables.
4. Complex data type in R
All complex numbers are called complex data types in the R language. An imaginary part is used to store numbers in complex data types.
5. Character data type in R
To store all alphabets and special characters we used character data type in R. It stores characters or strings (alphabets, symbols, and numbers). The best way to show that a value is a character data type in R is to wrap the value inside the double or single quote.
Following are some tasks that can be performed using data types in R programming:
Finding object data type in R
We used the class() function in the R language to check the data type of an object. We passed the object as an argument to the class() function for checking its data type.
Syntax:
A simple R program to find the data type of an object:
# Logical Data Type print(class(TRUE)) # Integer Data Type print(class(3L)) # Numeric Data Type print(class(5.5)) # Complex Data Type print(class(4+2i)) # Character Data Type print(class("06-09-2021"))
Output:

Verifying the data type in R
We can verify the data type of an object in R programming using the “is” prefix before the data type as a command.
Syntax:
A simple R program to verify if an object is of a certain datatype:
# Logical print(is.logical(TRUE)) # Integer print(is.integer(3L)) # Numeric print(is.numeric(5.5)) # Complex print(is.complex(4+2i)) # Character print(is.character("06-09-2021")) print(is.integer("a")) print(is.numeric(5+3i))
Output:
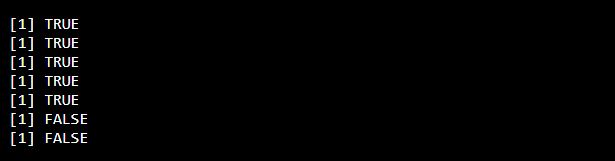
Data type conversion in R
We can convert the data type of any object in R language using the as.data_type() function. We convert the data type of an object in R programming using the “as” prefix before the data type as a command.
Syntax:
A simple R program to convert the data type of an object to another:
# Logical print(as.numeric(TRUE)) # Integer print(as.complex(3L)) # Numeric print(as.logical(5.5)) # Complex print(as.character(4+2i)) # Can't possible print(as.numeric("06-09-2021"))
Output:
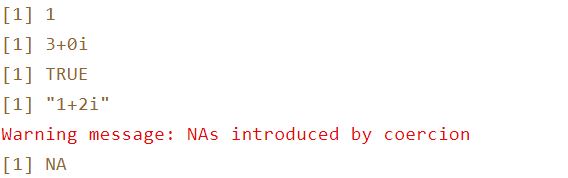