A switch statement in R is a selection control method that allows the value of an expression to change the flow control of program execution with help of search and map.
The switch statement in R is used to reserve of long if statement which compares a variable with the various integral values. This statement provides an easy way to dispatch the execution of different parts of code.
This statement permits a variable to be tested for the same against a list of values. The switch statement is a bit complex. Here are some key points to understand it:
- The string is matched to the listed cases, if expression type is a character string.
- First match element is used, in case of more than one match.
- Default case is not available in switch statement
- An unnamed case is used, if no case is matched.
There are two methods in which of the case is selected in R:
Based on Index
If the case is a value like a character vector, and the expression is checked to a number then the expression’s result is used as an index to select the case.
Based on Matching Value
When the case is like [“case_1″=”value1”] where the case and output values are included, then the expression is checked against the case value. If the case is matched, the corresponding value is the output.
The syntax for the switch statement is given below:
Flowchart for Switch Statement in R
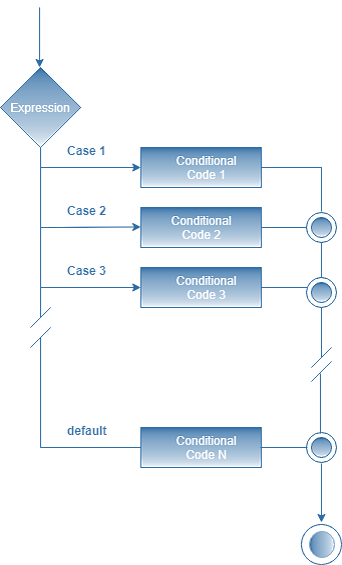
The following example will return the 3rd value as per the condition.
x <- switch( 3, "C++", "Python", "Java", "HTML" ) print(x)
Output:

The following example will return the value after adding as indexes for the given values.
a= 2 b = 2 y = switch( a+b, "C++", "Python", "HTML", "Java" ) print (y)
Output:

The following example will return the value after checking the key value of the given values.
y = "4" x = switch( y, "3"="C++", "6"="Python", "4"="Java", "2"="HTML" ) print (x)
Output:

The following example will return the value after combining the two values as indexes for the given values.
x= "4" y="1" a = switch( paste(x,y,sep=""), "9"="C++", "16"="Python", "14"="Java", "41"="HTML" ) print (a)
Output:

The following example applies the operations from given cases on the values.
y = "2" a=46 b=3 x = switch( y, "1"=cat("Addition=",a+b), "2"=cat("Subtraction =",a-b), "3"=cat("Division= ",a/b), "4"=cat("multiplication =",a*b) )
Output:
