The R function is a set of statements that are organized together to perform a particular task. R language facilitates a series of built-in functions and permits to use to create their own functions. The modular approach is used to perform tasks in functions.
Usually, functions are used to avoid repetition of the same task and mitigate the complexity. For understanding and maintaining the code, it technically segregates into smaller parts using the function. The keyword function is used to create the R function. A function must be
- Written to fetch a specified task.
- Can or cannot have arguments
- Contain a body in which code is written
- One or more output values may or may not return
The syntax of the R function is given below:
Elements of R Function
There are four components of function, which are as follows:
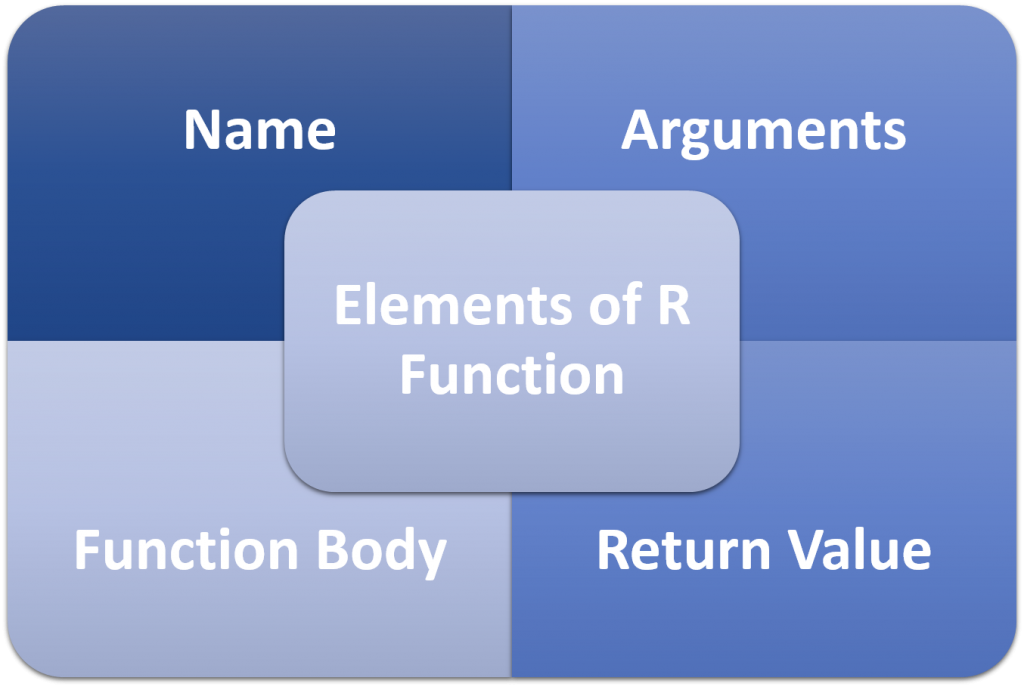
Name of function
The actual name of the function is the name of the function. The function is stored as an object with its name in R programming.
Arguments
An argument is a procurator in R programming. Arguments are optional means a function may or may not carry arguments in a function, these arguments can have default values too. The function is invoked when we pass a value to the argument.
Function Body
The function body carries a set of statements that define what the function is actually doing.
Return value
This is the last expression in the function body to be checked.
Types of R Function
Like the other languages, R has two types of functions, built-in function, and user-defined function. The built-in functions can directly call in the program without defining them. R programming also permits us to create our own functions.
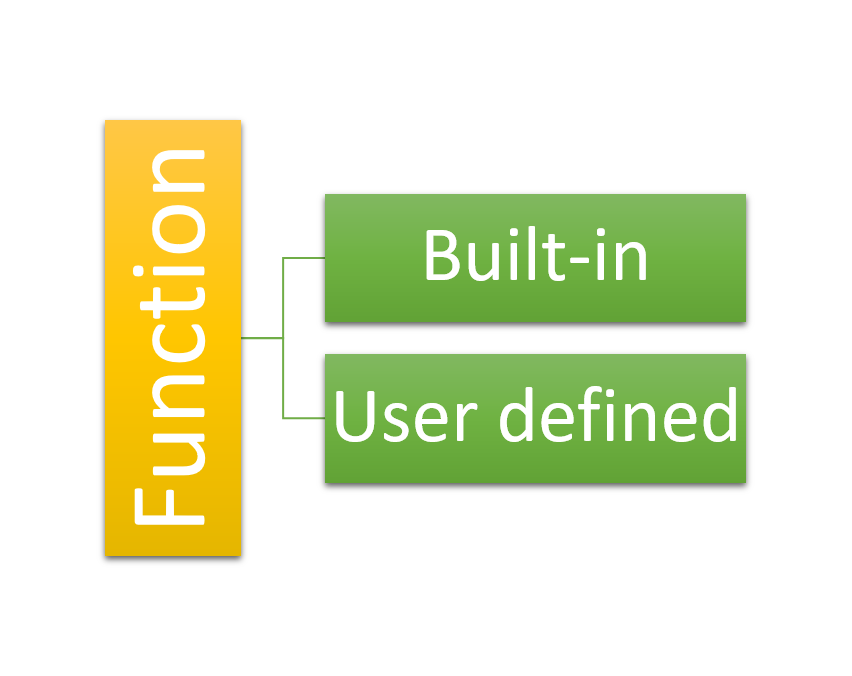
Built-in Function
The functions which are already defined or created in the language tool are known as built functions, we do not need to create the functions by the user, and these functions are built into an application. These functions simply can be access after calling by end-users. There are different types of built-in functions in R programming like max(), mean(), sum(x), seq() etc.
# Function to calculate Mean print(mean(16:76)) # Function to calculate Sum print(sum(16:76))
Output:

User-defined Function
We can create our own functions in R programming. User define functions fulfill the need of the user. We can use these functions like built-in functions once these functions are created.
fun <- function() { for(i in 1:8) { print(i^3) } } fun()
Output:
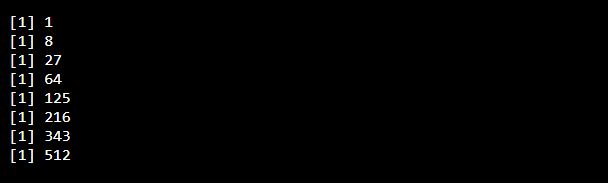
Following are four ways to call R Function:
Function calling with an argument
The function can be easily called by passing precisely the argument in the function. Have a look at the below example of how the function is called.
} fun <- function(a) { for(i in 1:a) { b <- i^2 print(b) } } # Calling the function with an argument. fun(5)
Output:

Function calling with no argument
In R, we can call a function without an argument in the following way:
fun <- function() { for(i in 1:8) { a <- i^2 print(a) } } # Calling the function without argument. fun()
Output:
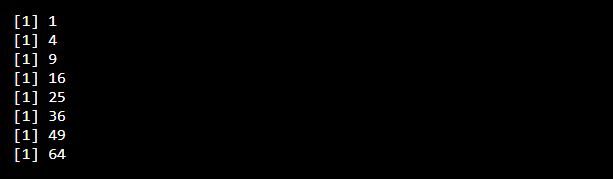
Function calling with Argument Values
The arguments can shift to a function call in the same series as defined in the function or can shift in a different series but assigned them to the names of the arguments.
fun <- function(a,b,c) { r <- a * b + c print(r) } # Calling function by position fun(10,56,25) # Calling function arguments name fun(a = 2, b = 5, c = 3)
Output

Function calling with default arguments
To take the default result, we designate the value to the arguments in the function definition and then we call the function without shifting argument. The default values get replaced if we pass the argument in the function definition.
fun <- function(a = 5, b = 24) { r <- a + b print(r) } # Calling the function without argument. fun() # Calling the function with new values fun(54,76)
Output
