Loop control statements are used to change the execution from its normal sequence. When execution leaves a scope all automatic objects are destroyed that were created in scope.
Following are some loop control statements in R language:
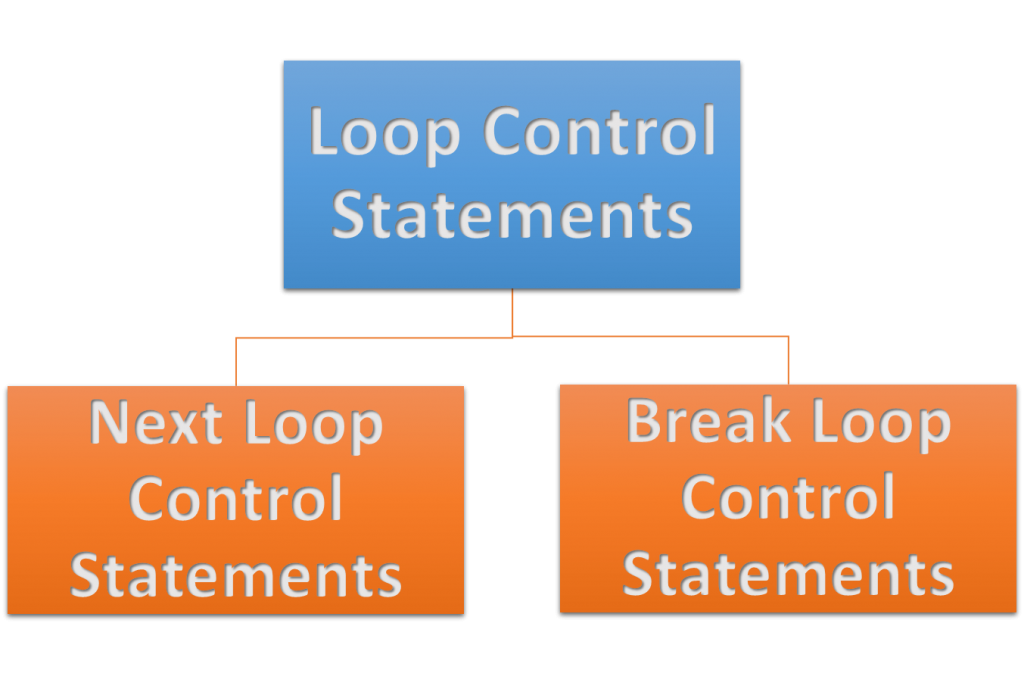
Break Loop Control Statements in R
To immediately exit from the loop and to break the execution of the loop break statement is used in R programming. In a nested loop, break statement control transfers to the outer loop and exits from the innermost loop only. It is very useful for handling program execution flow. It can be used with various loops like for, repeat, etc.
Following are some basic usage of break statement:
- When the break statement is inside the loop, the loop terminates immediately and program control resumes on the next statement after the loop.
- It is also used to terminate a case in the switch statement.
- We can also use break statement in else statement.
Following is the syntax for creating a break statement in R:
Flowchart of Break Statement in R
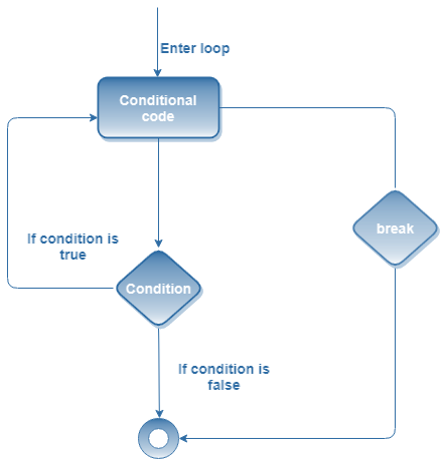
The following code will print “Tutorials Art” 8 times and then will break the execution.
a <- 3 repeat { print("Tutorials Art"); if(a >= 10) break a<-a+1 }
Output:
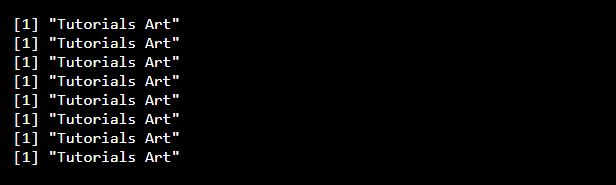
Next Loop Control Statements in R
To skip any remaining statements in the loop and continue executing the next statement is used in R programming. Simply, the next statement skips the current iteration of a loop without terminating it. The R parser skips the evaluation of the current iteration and starts the next iteration while the next statement is encountered.
This statement is mostly used with for loop and while loop. We can also use the next statement in the else statement.
Following is the basic syntax for creating the next statement in R:
Flowchart of Next Statement in R
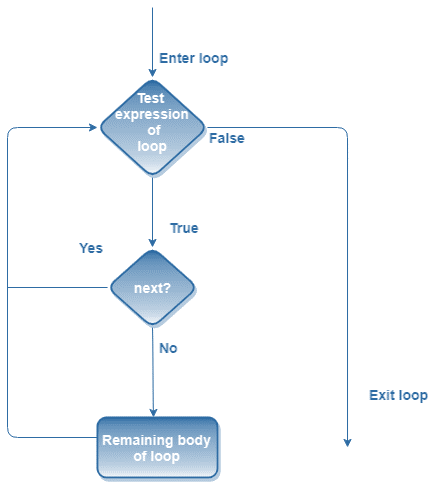
The following code will print counting from 1 to 4 using a repeat loop.
a <- 1 repeat { if(a == 8) break if(a == 5){ next } print(a) a <- a+1 }
Output:

The following code will print counting from 1 to 4 using a while loop.
a<-1 while (a < 8) { if(a==3) next print(a) a = a + 1 }
Output:

The following code will print counting from 1 to 10 using for loop.
a <- 1:5 for (v in a) { if (v == 3){ next } print(v) }
Output:
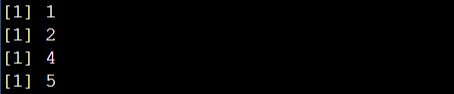
To learn about the above-mentioned loops click here.